Understanding AsyncData in Layout: A Guide for Developers
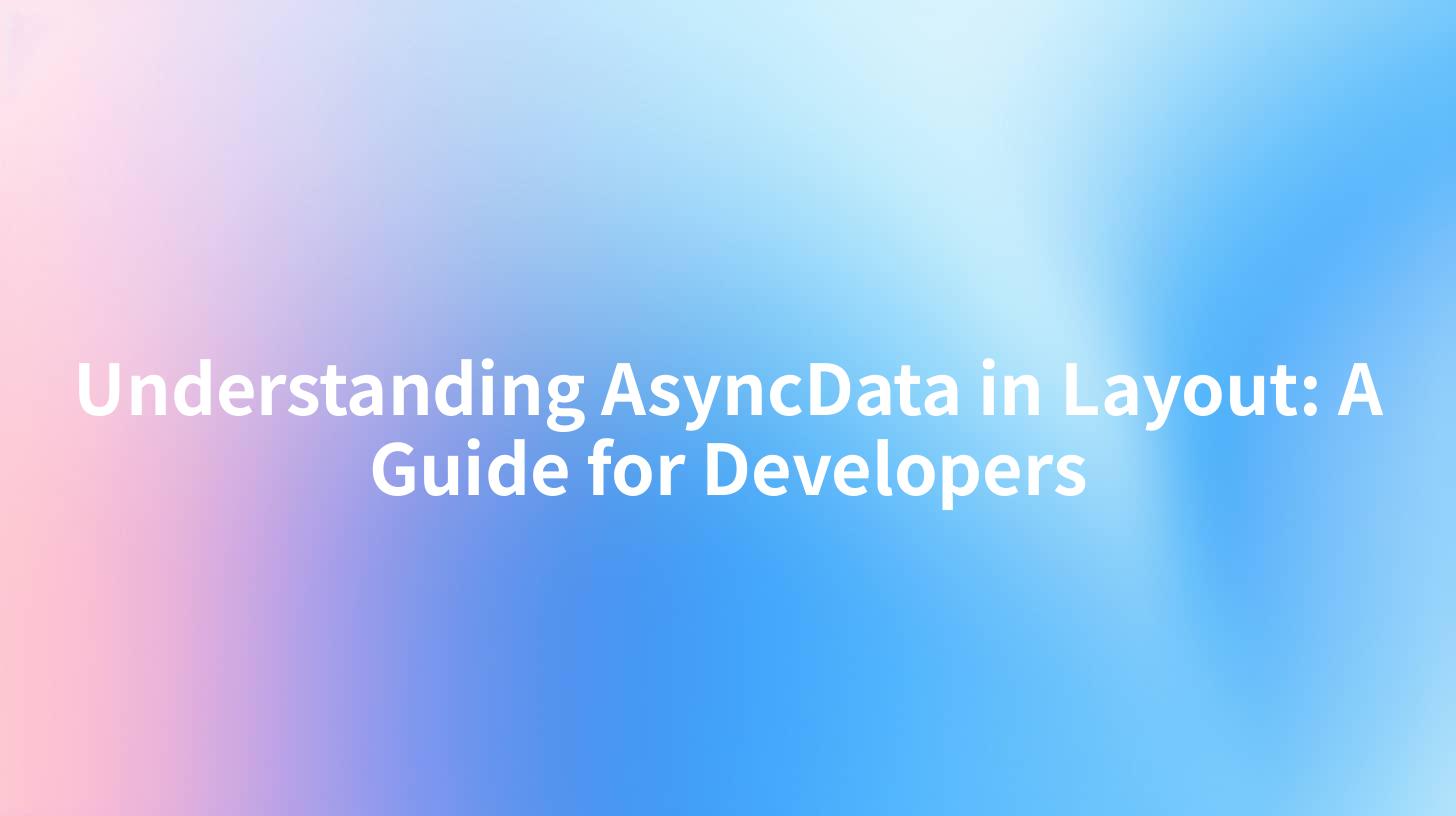
Open-Source AI Gateway & Developer Portal
Understanding AsyncData in Layout: A Guide for Developers
In the modern web development landscape, managing data efficiently is crucial for creating responsive and dynamic applications. Among various techniques available, leveraging AsyncData within layouts offers a powerful approach for handling data asynchronously while maximizing API capabilities. This article dives deep into understanding AsyncData, especially within the context of the truefoundry platform and API Open Platform, and emphasizes the importance of data format transformation. Let's unpack these concepts in detail.
What is AsyncData?
AsyncData is a method used in web frameworks to fetch data asynchronously and render it in the layout. This allows developers to load data not only when the component is mounted but also during the server-side rendering process. As a result, AsyncData improves the performance and user experience by reducing wait times for data retrieval.
Key Features of AsyncData
- Asynchronous Data Loading: AsyncData allows for the non-blocking retrieval of data, meaning that the user interface remains responsive while data is being fetched from an API or another source.
- Improved User Experience: By loading data asynchronously, components can render parts of the UI that do not depend on the incoming data, offering users a smoother interaction.
- Integration with APIs: Using AsyncData makes it easier to connect with various API endpoints, such as the ones offered by truefoundry and other API Open Platforms.
- Automatic State Management: Handling loading and error states becomes simple when using AsyncData as it inherently manages the states throughout the data fetching lifecycle.
API Interaction and AsyncData
When developing applications that rely heavily on API calls, the function of AsyncData becomes even more significant. Truefoundry, as an API Open Platform, provides extensive capabilities to manage API calls, ensuring developers can easily access and manipulate data from diverse sources.
How to Use API Calls with AsyncData
Here’s how you can properly implement API calls using AsyncData:
- Define API Endpoint: Choose your API endpoint from the Open API resource, ensuring you have the necessary permissions and keys to access the data.
- Configure AsyncData Function: Define an AsyncData function within your layout or component that will call the API endpoint.
- Handle Data Transformation: Often, the data received from APIs may not be in the format needed for your application. You will need to implement data format transformation to adapt the incoming data to your application's requirements.
Example Implementation
Here’s a basic example of how to implement an AsyncData function with an API call:
export default {
async asyncData({ $axios }) {
try {
const response = await $axios.$get('https://api.example.com/data')
return { data: response }
} catch (error) {
console.error('Error fetching data:', error)
return { data: null }
}
}
}
In this example, we use $axios
to make a GET request to our API. The data returned from the API call is then returned and can be accessed within your layout or component.
The Role of Data Format Transformation
Data format transformation is often a mandatory step before utilizing the data received from API calls. Here’s why:
- Standardization: API responses can greatly vary in structure. Transforming data ensures that the format aligns with your application’s expected structure.
- Optimization: By only fetching the necessary fields and organizing the data accordingly, you can enhance performance, reducing the load time.
- Error Reduction: Proper transformation reduces the chances of runtime errors caused by unforeseen data structures or types.
Utilizing Truefoundry for AsyncData Implementation
Truefoundry is an excellent resource for deriving API interactions when implementing AsyncData. Its AI-powered API Open Platform simplifies the integration of various data sources while ensuring data stays consistent and secure across environments.
Step-by-Step Implementation with Truefoundry
- Register for Access: Sign up for Truefoundry and request API access to necessary services.
- Obtain API Keys: Once your application is created, generate the required API keys for authentication purposes.
- Set Up AsyncData Calls: With your keys in hand, configure your AsyncData calls as mentioned earlier, ensuring to structure your requests to suit API requirements.
- Transform Data Between Formats: Depending on your application needs, set up functions that will handle the transformation of data formats received from the API calls.
Example of API Call with Truefoundry
This is a sample code demonstrating how to make an API call to Truefoundry's service using AsyncData:
export default {
async asyncData({ $axios }) {
const apiKey = process.env.TRUEFOUNDRY_API_KEY // Storing API key securely
try {
const response = await $axios.$get('https://api.truefoundry.com/data', {
headers: {
'Authorization': `Bearer ${apiKey}`
}
})
const transformedData = response.map(item => ({
id: item._id,
name: item.attributes.name,
// additional transformations here
}));
return { data: transformedData }
} catch (error) {
console.error('Error fetching data from Truefoundry:', error)
return { data: [] }
}
}
}
In this code snippet, we've incorporated an API call to Truefoundry, transformed the data for optimal usage, and handled potential errors gracefully.
Best Practices for Implementing AsyncData in Layout
When working with AsyncData, there are best practices that can enhance the effectiveness and performance of your applications:
- Error Handling: Always include error handling in your AsyncData methods to gracefully handle issues that arise during API calls.
- Loading States: Implement loading indicators to inform users that data is being fetched. This can be accomplished using boolean flags managed within your state.
- Caching: Consider caching strategies for data that doesn't change frequently. This can significantly improve performance and reduce unnecessary API calls.
- Organizing Data: Keep your data organized and structured, particularly when transforming data formats to maintain readability and reduce complexity.
- Testing: Regularly test your AsyncData implementations to catch issues early on, ensuring that any transformation problems or API changes don’t disrupt user interaction.
Summary
AsyncData in layout represents a potent tool for developers looking to manage data effectively in modern web applications. By utilizing tools such as the truefoundry API Open Platform, developers can streamline data retrieval, ensure effective formatting through transformation, and improve overall user experiences.
By following the practices outlined in this guide and leveraging the power of APIs, your applications can achieve peak performance and adaptability.
Future Prospects
The future of web development increasingly leans towards asynchronous operations, particularly with the advent of technologies like serverless architecture and edge computing. Leveraging AsyncData will continue to play a vital role in these advancements, ensuring that developers can keep up with the demands of responsive, data-driven applications.
Table: Advantages of Using AsyncData
Advantage | Description |
---|---|
Improved Performance | Non-blocking data retrieval enhances UI responsiveness |
User Experience Enhancement | Users receive immediate feedback during data loading |
API Integration Facilitation | Simplifies interaction with diverse APIs |
State Management | Automatically handles different data states across the app |
Flexibility in Data Handling | Allows for quick adaptations to changing data formats |
APIPark is a high-performance AI gateway that allows you to securely access the most comprehensive LLM APIs globally on the APIPark platform, including OpenAI, Anthropic, Mistral, Llama2, Google Gemini, and more.Try APIPark now! 👇👇👇
As a developer, embracing AsyncData opens up new avenues in application design and management, resulting in robust and high-performing products. By understanding and applying these concepts effectively, you can ensure your applications remain at the forefront of web development trends.
🚀You can securely and efficiently call the 通义千问 API on APIPark in just two steps:
Step 1: Deploy the APIPark AI gateway in 5 minutes.
APIPark is developed based on Golang, offering strong product performance and low development and maintenance costs. You can deploy APIPark with a single command line.
curl -sSO https://download.apipark.com/install/quick-start.sh; bash quick-start.sh
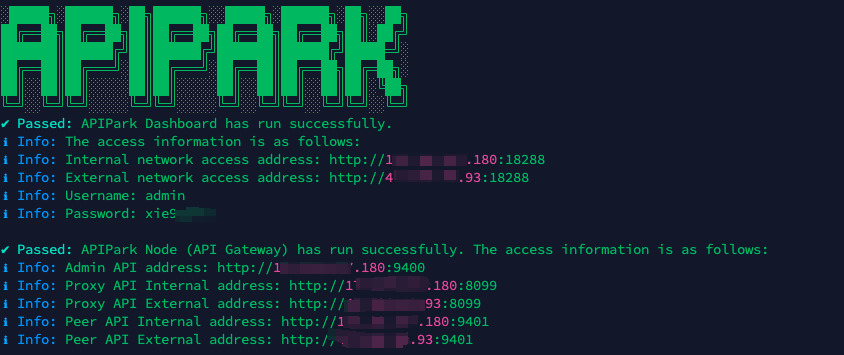
In my experience, you can see the successful deployment interface within 5 to 10 minutes. Then, you can log in to APIPark using your account.
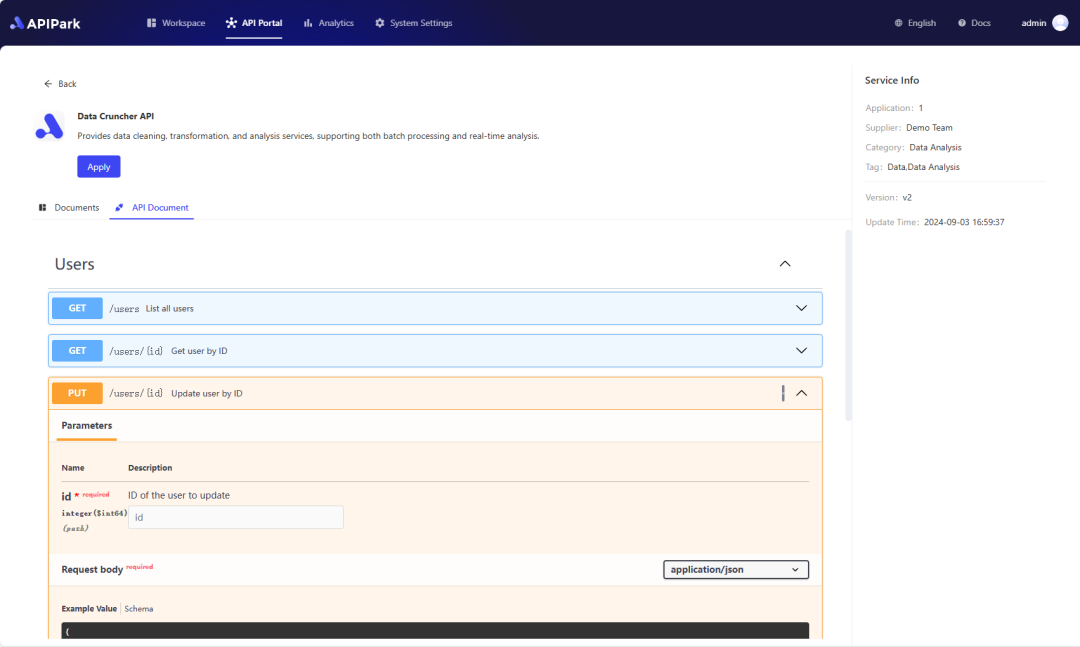
Step 2: Call the 通义千问 API.
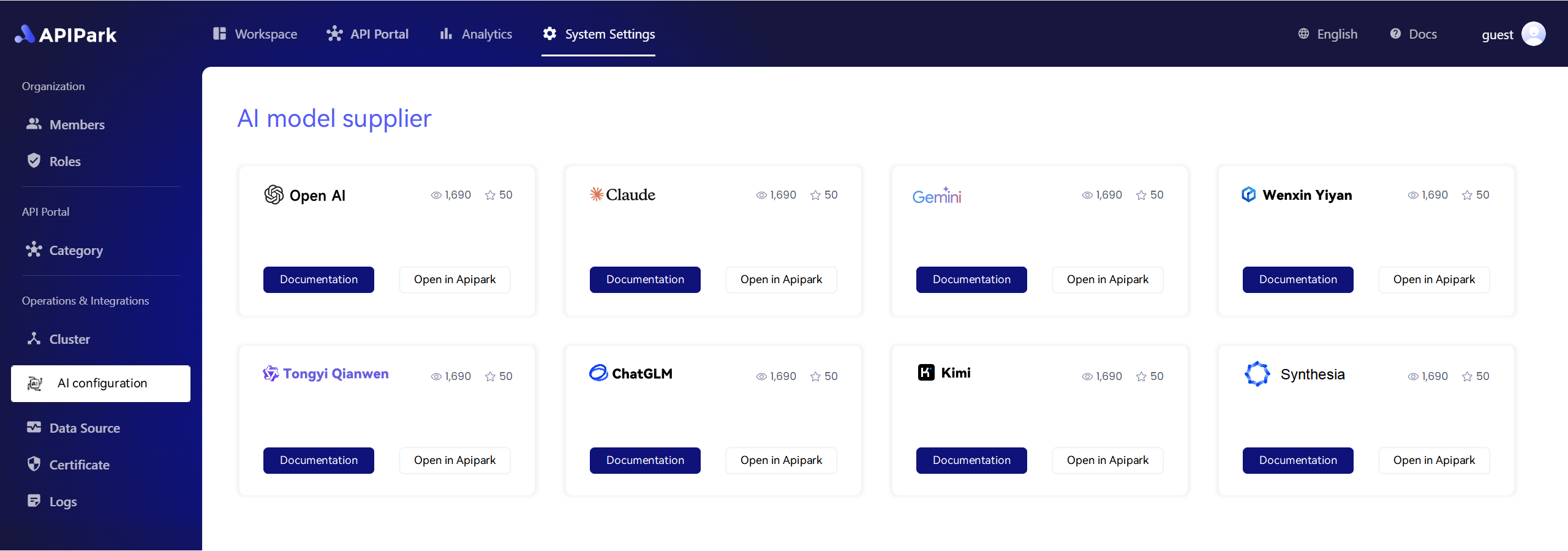