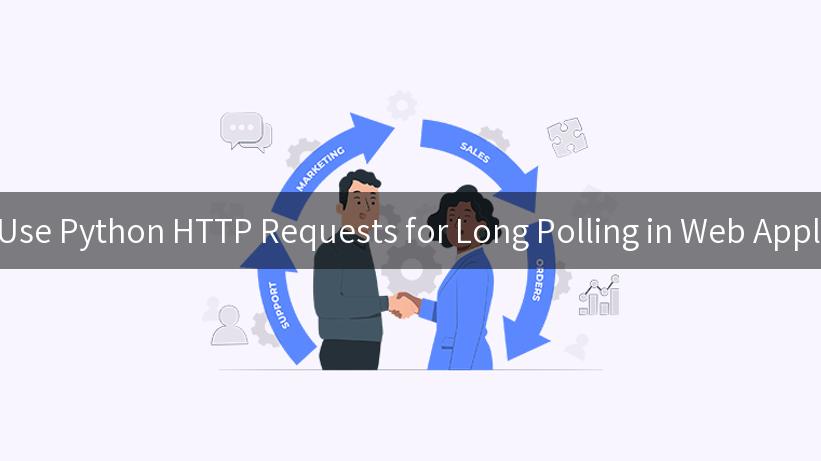
Long polling is a technique used in web applications to maintain a persistent connection between the client and server. It’s particularly useful for real-time applications where updates need to be pushed from the server whenever data changes. In this article, we’ll explore how to implement long polling using Python’s HTTP request library, which will allow you to send requests effectively while managing the connection lifecycle.
Introduction to Long Polling
Long polling is a variation of the polling technique. In traditional polling, the client sends regular requests to the server at fixed intervals to check for any updates. However, this can lead to frequent unnecessary requests when there are no updates available.
In contrast, long polling involves the client making a request to the server and the server holding that request open until there is new information available. Once the server has an update, it responds to the request, and the client can then immediately send a new request to wait for more updates. This results in fewer requests and reduced server load while providing a more efficient user experience.
Advantages of Long Polling
- Real-Time Updates: Clients receive updates from the server as soon as they occur, leading to a more interactive experience for users.
- Reduced Bandwidth Usage: By maintaining a single connection for longer durations, long polling can lower the bandwidth consumed by your application.
- Simplified Implementation: Compared to WebSockets, long polling can be easier to implement with existing HTTP-based APIs.
Understanding Python’s HTTP Requests for Long Polling
Python provides several libraries that simplify making HTTP requests, with the most popular being requests
. This section will demonstrate how to use the requests
library effectively for long polling scenarios.
Installing the Requests Library
First, ensure you have the necessary library installed. If it is not already installed, you can install it via pip:
pip install requests
Example Structure for Long Polling
Below is a sample Python script demonstrating how to implement long polling using HTTP requests. The script continuously sends requests to a server and expects updates containing new messages.
import requests
import time
SERVER_URL = "https://api.example.com/long-poll"
LONG_POLL_TIMEOUT = 30 # Maximum time to wait for data in seconds
def long_poll():
while True:
try:
# Send an HTTP GET request to the server, specifying a timeout
response = requests.get(SERVER_URL, timeout=LONG_POLL_TIMEOUT)
response.raise_for_status() # Raise an error for bad responses
# Process the response data (assuming JSON format)
data = response.json()
print("New data received:", data)
except requests.exceptions.Timeout:
print("Request timed out. Retrying...")
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
# Wait before sending another request
time.sleep(1)
if __name__ == "__main__":
long_poll()
How It Works
- Continuous Loop: The
while True:
loop ensures that the request is sent perpetually.
- HTTP GET Request: The
requests.get(SERVER_URL, timeout=LONG_POLL_TIMEOUT)
line sends a long poll request to the specified server.
- Response Handling: Once the server responds, we check for errors and process the received data.
- Delay Between Requests: The script includes a slight delay (controlled by
time.sleep(1)
) before making the next request.
Challenges with Long Polling
Though effective, long polling may have its challenges that developers must consider:
- Server Load: Each open connection consumes server resources, potentially leading to scalability challenges.
- Timeout Management: If the server takes too long to respond, requests may timeout, necessitating robust error handling.
- Network Latency: High latency can lead to slower updates received by the client.
Improving Long Polling Performance
To mitigate some of these challenges, you can implement optimizations like:
- Backoff Strategies: Implement strategies that gradually increase the wait time between retries after timeouts or errors.
- Connection Reuse: Wherever possible, reuse existing connections to reduce overhead.
- Monitoring and Metrics: Implement monitoring solutions to track the performance of your long polling implementation.
Integrating AI Security with Long Polling
When it comes to security, especially in modern applications that incorporate AI components, it’s vital to understand the API gateway aspect along with IBM API Connect. Security measures should be integrated to ensure that your long polling connections are secure.
API Gateway Features
Using an API gateway like IBM API Connect offers several advantages:
- Security Features: Provides tools for authentication, such as OAuth and JWT, to secure your API endpoints from unauthorized access.
- Rate Limiting: Helps manage the load on your backend servers by restricting the number of requests a user can make.
- Parameter Rewrite/Mapping: Allows altering the parameters coming into your API to conform to the expected formats, enhancing interoperability.
Securing Long Polling with AI
Integrating AI security features can enhance the data protection aspect of your long polling implementation. For example, anomaly detection algorithms could monitor for unusual traffic patterns indicative of attacks or hacking attempts.
Setting Up Your Environment
To illustrate a more comprehensive setup, let’s take a look at using IBM API Connect in conjunction with long polling.
- Create an API in IBM API Connect: Set up your API services and configure routes accordingly.
- Apply Security Policies: Use built-in policies to secure your APIs, enforce authentication, and monitor traffic.
- Deploy Your Application: Make your long polling service accessible via the API gateway you configured.
Conclusion
In this article, we have explored how to use Python’s HTTP requests effectively for implementing long polling in web applications. We covered the essentials of long polling, challenges you might face, and how to integrate security practices using API gateways such as IBM API Connect.
Long polling is a powerful traditional solution for real-time updates, providing applications with the efficiency of notification delivery while consuming fewer resources than standard polling techniques. By combining Python’s capabilities with AI security and API management, developers can create robust, scalable web applications that meet the demands of users.
Summary Table
Feature |
Long Polling |
Benefits |
Connection Type |
Persistent |
Real-time updates |
Client Request Frequency |
Less frequent |
Reduced server and network load |
Server Resources |
More utilized |
Improved efficiency |
Error Handling |
Necessary |
Reliability in communication |
Feel free to adapt the code and examples as per your specific application requirements. Happy coding!
APIPark is a high-performance AI gateway that allows you to securely access the most comprehensive LLM APIs globally on the APIPark platform, including OpenAI, Anthropic, Mistral, Llama2, Google Gemini, and more.Try APIPark now! 👇👇👇
This article serves as a foundational guide for implementing long polling in your applications. For real-world applications, incorporate monitoring solutions, testing frameworks, and security mechanisms for a robust deployment.
🚀You can securely and efficiently call the gemni API on APIPark in just two steps:
Step 1: Deploy the APIPark AI gateway in 5 minutes.
APIPark is developed based on Golang, offering strong product performance and low development and maintenance costs. You can deploy APIPark with a single command line.
curl -sSO https://download.apipark.com/install/quick-start.sh; bash quick-start.sh
In my experience, you can see the successful deployment interface within 5 to 10 minutes. Then, you can log in to APIPark using your account.
Step 2: Call the gemni API.