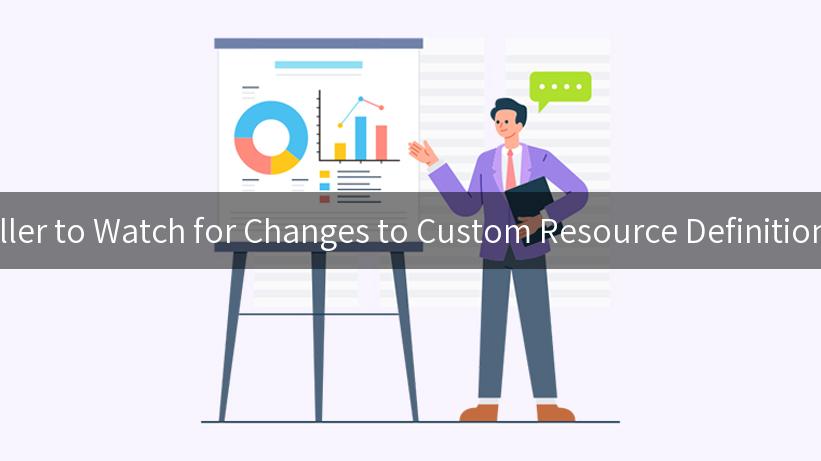
Kubernetes has become the de facto standard for container orchestration, providing developers with the necessary tools to manage applications in a cloud-native environment. A critical feature of Kubernetes is its ability to extend the API with Custom Resource Definitions (CRDs). CRDs enable developers to define custom resources, thereby allowing Kubernetes to manage and interact with these resources seamlessly.
In this article, we will explore the process of implementing a controller to watch for changes to CRDs in Kubernetes. We will cover how to leverage APIPark, IBM API Connect, and the API Developer Portal while looking at various best practices and implementation strategies. Along the way, we will also use diagrams and code snippets to illustrate key concepts effectively.
Understanding Custom Resource Definitions (CRDs)
Before diving into implementing a controller, let’s briefly understand what CRDs are and how they work. In Kubernetes, a CRD is an extension mechanism that allows users to add their own API objects to the Kubernetes API.
For example, if we wanted to manage a new type of object—say, a “Database” resource—using a CRD, we would define the specifications for that resource, like so:
apiVersion: apiextensions.k8s.io/v1
kind: CustomResourceDefinition
metadata:
name: databases.example.com
spec:
group: example.com
versions:
- name: v1
served: true
storage: true
schema:
openAPIV3Schema:
type: object
properties:
spec:
type: object
properties:
size:
type: string
scope: Namespaced
names:
plural: databases
singular: database
kind: Database
shortNames:
- db
Benefits of Using CRDs
- Flexibility: CRDs allow you to define new resource types that meet your application’s needs.
- Integration: They integrate seamlessly with existing Kubernetes tools and workflows.
- Extensibility: You can extend the Kubernetes API to include domain-specific logic.
Building a Controller to Watch for Changes to CRDs
When working with CRDs, it is common to require a controller that can respond to changes in the CRD’s state. A controller is essentially a loop that watches Kubernetes resources and performs actions when they change.
Step 1: Set Up Your Go Environment
Most Kubernetes controllers are written in Go. To start, ensure you have Go installed on your machine. If it’s not installed yet, follow the official Go installation guide.
Next, create a new directory for your project:
mkdir crd-controller
cd crd-controller
go mod init crd-controller
Step 2: Install Necessary Libraries
You will need client-go and controller-runtime to interact with Kubernetes:
go get k8s.io/client-go@kubernetes-1.20.0
go get sigs.k8s.io/controller-runtime@v0.8.0
Step 3: Implement the Controller Logic
Below is a basic implementation of a controller that watches for changes to the Database CRD:
package main
import (
"context"
"fmt"
"os"
apiv1 "k8s.io/api/core/v1"
metav1 "k8s.io/apimachinery/pkg/apis/meta/v1"
"k8s.io/client-go/kubernetes"
"k8s.io/client-go/tools/clientcmd"
"sigs.k8s.io/controller-runtime/pkg/client"
"sigs.k8s.io/controller-runtime/pkg/manager"
)
func main() {
configPath := os.Getenv("KUBECONFIG")
config, err := clientcmd.BuildConfigFromFlags("", configPath)
if err != nil {
panic(err.Error())
}
mgr, err := manager.New(config, manager.Options{})
if err != nil {
panic(err.Error())
}
dbClient := mgr.GetClient()
watchDatabase(dbClient)
}
func watchDatabase(c client.Client) {
for {
var databases apiv1.CustomResourceDefinitionList
err := c.List(context.Background(), &databases, &client.ListOptions{})
if err != nil {
fmt.Println("Error fetching Database CRDs:", err)
continue
}
for _, db := range databases.Items {
fmt.Printf("Database found: %s\n", db.Name)
}
// Implement more logic as needed here...
}
}
Step 4: Deploying Your Controller
Once you have your controller implemented, it can be deployed to your Kubernetes cluster as part of a pod. Below is an example deployment configuration you may use:
apiVersion: apps/v1
kind: Deployment
metadata:
name: db-controller
spec:
replicas: 1
selector:
matchLabels:
app: db-controller
template:
metadata:
labels:
app: db-controller
spec:
containers:
- name: db-controller
image: YOUR_IMAGE_HERE
env:
- name: KUBECONFIG
value: /path/to/your/kubeconfig
Integrating with APIPark and IBM API Connect
APIPark is a robust platform that provides centralized management for APIs. When using CRDs, especially with external APIs, you can use APIPark to bridge the gap. By leveraging APIPark, you can allow applications that manage CRDs to communicate effectively with external services.
Benefits of Integrating APIPark
- API Gateway: APIPark acts as an API gateway, providing a centralized access point to your CRD-managed services.
- Analytics and Logging: With APIPark, you can track API consumption via dashboards and logging features.
- Security: You can manage access policies and ensure data security through API management features.
Using IBM API Connect
When integrating with IBM API Connect, you’ll benefit from its features for exposing APIs securely and managing them efficiently. The integration allows Kubernetes services defined through CRDs to be consumed easily.
Example Diagram
Here is a simplified diagram illustrating how the components interact:
+---------------------+
| Kubernetes |
| |
| +-----------------+ |
| | CRD Controller | |
| +-----------------+ |
| | |
+---------|-----------+
|
v
+---------------------+
| APIPark |
| |
| +-----------------+ |
| | API Gateway | |
| +-----------------+ |
| | |
+---------|-----------+
|
v
+---------------------+
| External Services |
| |
+---------------------+
Detailed Logging and Monitoring
To ensure that your controller runs smoothly and interactions with CRDs occur without issues, implementing detailed logging is crucial. Kubernetes provides mechanisms for logging, but you can enhance this with integrated tools like ELK Stack or Prometheus. By doing this, you can monitor the performance and diagnose any anomalies.
Example: Detailed Logging with Go
Below is a simplified logging structure you can integrate into your Kubernetes controller:
import (
"k8s.io/klog/v2"
)
func logDatabaseEvent(db apiv1.CustomResourceDefinition) {
klog.Infof("Database event - Name: %s, Status: %s", db.Name, db.Status)
}
func watchDatabase(c client.Client) {
for {
var databases apiv1.CustomResourceDefinitionList
err := c.List(context.Background(), &databases, &client.ListOptions{})
if err != nil {
logDatabaseEvent(db) // Logs the database event
continue
}
// Log other events as needed...
}
}
APIPark is a high-performance AI gateway that allows you to securely access the most comprehensive LLM APIs globally on the APIPark platform, including OpenAI, Anthropic, Mistral, Llama2, Google Gemini, and more.Try APIPark now! 👇👇👇
This enriches the context of your CRD controller to watch for changes effectively.
Conclusion
Implementing a controller to watch for changes in Custom Resource Definitions (CRDs) is a fundamental aspect of modern Kubernetes practices. This guide walked you through creating a simple controller in Go and discussed integrating it with APIPark and IBM API Connect for more substantial API management capabilities.
By understanding how to leverage CRDs and build responsive controllers, developers can create robust, dynamic applications that can scale and adapt to changing requirements quickly. Coupling this knowledge with the powerful features offered by platforms like APIPark consolidates API management, allowing enterprises to innovate effectively.
References
- Kubernetes Official Documentation
- APIPark Documentation
- IBM API Connect
This knowledge will set a solid foundation for developing Kubernetes applications that make the most of CRDs and their dynamic capabilities in a cloud-native ecosystem.
🚀You can securely and efficiently call the claude(anthropic) API on APIPark in just two steps:
Step 1: Deploy the APIPark AI gateway in 5 minutes.
APIPark is developed based on Golang, offering strong product performance and low development and maintenance costs. You can deploy APIPark with a single command line.
curl -sSO https://download.apipark.com/install/quick-start.sh; bash quick-start.sh
In my experience, you can see the successful deployment interface within 5 to 10 minutes. Then, you can log in to APIPark using your account.
Step 2: Call the claude(anthropic) API.