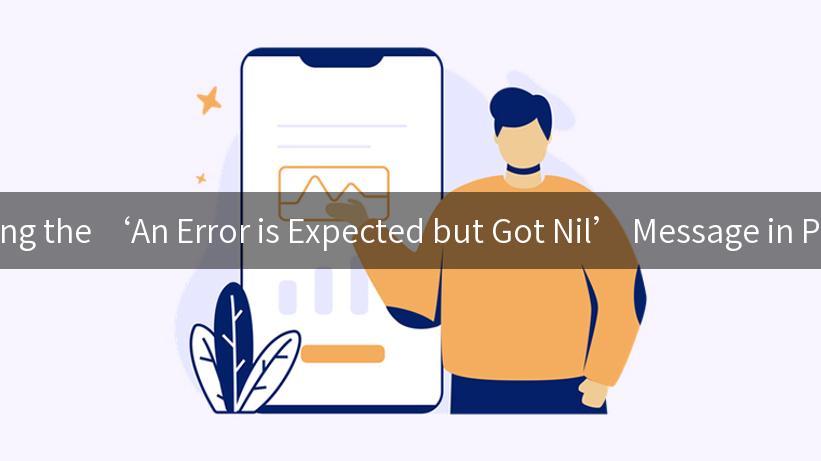
In the realm of software development, error handling and message clarity play a crucial role in ensuring robust, maintainable, and user-friendly applications. One common message that developers encounter is the phrase, “An error is expected but got nil.” This message can be especially haunting for programmers blocked by seemingly trivial mistakes that often lead to profound implications in the program’s logic and performance. In this article, we will dissect the aspects surrounding this message, focusing on contexts where it appears, its implications on API management, and some best practices for error handling.
The Basics of Error Handling
In programming, an error is a condition that disrupts the normal flow of a program’s execution. Errors can happen for various reasons: incorrect data input, unexpected user actions, or even misconfigurations in the environment (like API settings in a cloud platform). Robust error handling ensures that such issues are managed gracefully, enhancing user experience and debugging efficiency.
Here’s a simple illustration of the concept of an error in programming through a pseudocode example:
function fetchData(apiUrl, apiKey):
if apiUrl is invalid or apiKey is missing:
return "An error is expected but got nil"
data = callApi(apiUrl, apiKey)
return data
In this pseudocode, if the apiUrl
is invalid, or the apiKey
is not provided, the function returns an error message rather than proceeding with the API call, demonstrating fundamental error-handling principles.
The ‘Nil’ Concept in Programming Languages
The term “nil” is often used interchangeably with “null” in programming languages, and it signifies the absence of a value. This concept is critical, especially in languages like Go, Ruby, and others where nil or null values can impact logic flow and application behavior.
The Error Message Analysis
The message “An error is expected but got nil” is indicative of a situation in which a function or method was anticipated to return an error, but instead, it yielded nil
. This situation often puzzles developers, leading to wasted time in debugging and better understanding of the underlying logic.
Possible Scenarios
-
Improper Error Handling: The function might be designed to handle certain failures but returns nil instead of the expected error. This oversight can lead to cascading failures in code where subsequent operations depend on valid responses.
-
Misconfiguration in APIs: In API management, especially when using platforms like the AI Gateway, Cloudflare, or API Open Platforms, configuration issues can occasionally lead to misreported state or errors, leading developers to encounter nil responses where errors are expected.
-
Asynchronous Operations: In asynchronous environments, the race conditions might lead to situations where the expected error condition is bypassed, resulting in nil instead.
Best Practices for Managing API Errors
When working with APIs, especially using services such as the AI Gateway or Cloudflare, it’s crucial to handle potential errors robustly. Here’s a table summarizing best practices for API error management:
Best Practice |
Description |
Implement Comprehensive Logging |
Log every API interaction, noting response data, headers and errors to understand failure states better. |
Utilize Status Codes |
Use appropriate HTTP status codes to reflect the kind of errors that are occurring during API calls. |
Employ Retry Logic |
For transient errors or specific api calls, implement retry logic to manage heavy-load conditions or brief downtimes. |
Configuration Validation |
Ensure all API keys (like AKSK) and other configurations are validated before calling end-points to minimize nil responses. |
Response Validation |
Always validate responses from API calls, handling nil cases gracefully to avoid cascading failures in the system. |
Implementing Error Handling with Authentication
When interfacing with APIs security measures like Basic Auth, AKSK, or JWT tokens play an important role. If these methods of authentication are mismanaged, a developer may face silent failures leading to the error message “an error is expected but got nil”.
Example of API Call with Error Handling
Here’s a practical example of how a well-structured API request might look, ensuring we handle errors correctly:
curl --location 'https://api.example.com/data' \
--header 'Content-Type: application/json' \
--header 'Authorization: Bearer your_jwt_token' \
--data '{
"query": "fetch_data"
}'
if [ $? -ne 0 ]; then
echo "An error is expected but got nil during API call"
exit 1
fi
In this bash script, we’re performing an API call and checking for errors using $?
, which captures the exit status of the last command. If an error occurs, it outputs the relevant message, assisting in tracing back potential issues.
Dealing with Errors in Modern Development Practices
In the context of modern development practices, adopting principles such as Continuous Integration/Continuous Deployment (CI/CD) can significantly reduce the occurrences of unexpected nil values. Automated testing setups will help catch these issues earlier in the development cycle.
Employing tools and platforms that provide more comprehensive error reporting and metrics, like logging frameworks or the built-in debugging tools from cloud providers, can vastly improve debugging experiences in programming.
APIPark is a high-performance AI gateway that allows you to securely access the most comprehensive LLM APIs globally on the APIPark platform, including OpenAI, Anthropic, Mistral, Llama2, Google Gemini, and more.Try APIPark now! 👇👇👇
Conclusion
Understanding and appropriately handling the message “an error is expected but got nil” can be pivotal in ensuring a smooth programming workflow, especially when integrating varying APIs through platforms like the AI Gateway or using authentication mechanisms like AKSK. By implementing solid error handling strategies and logging practices, developers can reduce confusion and increase the maintainability of their code.
Through this extensive exploration of error handling, we’ve established crucial links among error messages, API management, and best practices. By adopting these practices, developers can create resilient applications that handle errors gracefully, ultimately leading to a better user experience and easier maintenance.
In the world of programming, clarity is key, and the proper handling of errors — including understanding when nil arises where an error is anticipated — remains a fundamental skill every programmer should cultivate.