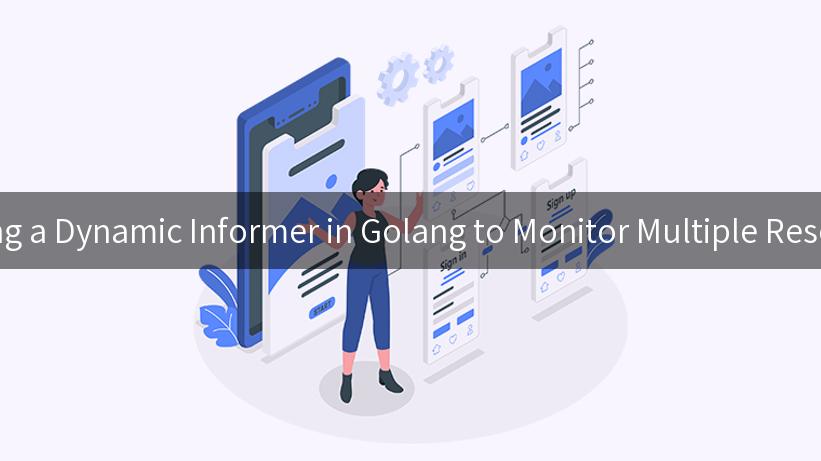
In the modern world of development, especially in the realm of cloud computing and microservices, monitoring varied resources efficiently is paramount. A dynamic informer in Golang allows developers to establish real-time monitoring mechanisms for multiple resources, ensuring more accurate and timely data handling. This article aims to delve deep into the construction of a dynamic informer, integrating aspects such as API security, AWS API Gateway, AI Gateway, and parameter rewrite/mapping.
Table of Contents
- Introduction
- Why Use a Dynamic Informer?
- Key Concepts and Technologies
- API Security
- AWS API Gateway
- AI Gateway
- Parameter Rewrite/Mapping
- Building the Dynamic Informer
- Setting Up the Environment
- Creating the Golang Project
- Coding the Dynamic Informer
- Structuring Your Code
- Implementing API Calls with AWS API Gateway
- Testing the Dynamic Informer
- Conclusion
- References
1. Introduction
The complexity of modern applications often necessitates monitoring multiple resources dynamically. A dynamic informer in Golang enables developers to listen for changes across various resources, facilitating better response mechanisms and more informed decision-making. With the introduction of API security aspects and integration with services like AWS API Gateway and AI Gateway, creating a robust yet flexible dynamic informer becomes indispensable for today’s development practices.
2. Why Use a Dynamic Informer?
Using a dynamic informer offers several advantages:
– Real-time Monitoring: Provides immediate feedback on resource changes.
– Scalability: Easily scalable to monitor multiple resources simultaneously.
– Custom Notifications: Tailor notifications for specific resource changes.
– Efficiency: Reduces overhead by monitoring changes rather than pulling data.
These aspects ensure that the system remains accurate, secure, and responsive to the needs of the organization.
3. Key Concepts and Technologies
API Security
API security is critical in ensuring that data transmitted over networks is not compromised. This encompasses a range of practices such as authentication, authorization, encryption, and protection against attacks.
AWS API Gateway
AWS API Gateway provides a robust solution for creating, deploying, and managing secure APIs at any scale. It allows you to build and integrate API services easily, streamlining the process of resource monitoring.
AI Gateway
AI Gateway enables integration with various AI services, offering the ability to leverage intelligent features and enhance application functionality. By monitoring resources alongside AI capabilities, businesses can gain deeper insights.
Parameter Rewrite/Mapping
Parameter rewrite/mapping enables the customization of how parameters are passed between different services. When constructing a dynamic informer, maintaining consistency in how data is handled across services is essential.
4. Building the Dynamic Informer
Setting Up the Environment
To create a dynamic informer in Golang, make sure you have the following installed:
– Go programming language
– AWS CLI for managing APIs
– A code editor like Visual Studio Code
Creating the Golang Project
Create a new folder for your Golang project and initialize a new module.
mkdir dynamic-informer
cd dynamic-informer
go mod init dynamic-informer
APIPark is a high-performance AI gateway that allows you to securely access the most comprehensive LLM APIs globally on the APIPark platform, including OpenAI, Anthropic, Mistral, Llama2, Google Gemini, and more.Try APIPark now! 👇👇👇
5. Coding the Dynamic Informer
Structuring Your Code
Let’s structure our code files to manage the informer effectively.
File Name |
Description |
main.go |
The entry point of the application. |
informer.go |
Contains the logic for the dynamic informer. |
api_client.go |
Handles API interactions. |
config.go |
Manages configuration settings for API gateways. |
Implementing API Calls with AWS API Gateway
Here’s an example of how to perform an API call using the AWS API Gateway. The following code illustrates a basic API call using Golang.
package main
import (
"bytes"
"fmt"
"net/http"
"os"
)
func callAWSApi() {
url := "https://your-api-id.execute-api.region.amazonaws.com/your-stage"
payload := []byte(`{"message": "Hello from Dynamic Informer!"}`)
req, err := http.NewRequest("POST", url, bytes.NewBuffer(payload))
if err != nil {
fmt.Println(err)
return
}
req.Header.Set("Content-Type", "application/json")
req.Header.Set("Authorization", "Bearer " + os.Getenv("API_TOKEN"))
client := &http.Client{}
resp, err := client.Do(req)
if err != nil {
fmt.Println(err)
return
}
defer resp.Body.Close()
fmt.Println("Response Status:", resp.Status)
}
func main() {
callAWSApi()
}
Ensure you’ve set the API_TOKEN
environment variable for authorization when calling your API.
6. Testing the Dynamic Informer
Once you’ve coded your dynamic informer, it’s essential to conduct thorough testing. Use tools like Postman or cURL to simulate requests to your API Gateway, observing how your dynamic informer responds to events or changes in resource states.
Sample Test Command
Here’s a sample command to test your API:
curl --location 'https://your-api-id.execute-api.region.amazonaws.com/your-stage' \
--header 'Content-Type: application/json' \
--header 'Authorization: Bearer your_token' \
--data '{
"message": "Testing Dynamic Informer!"
}'
7. Conclusion
Building a dynamic informer in Golang to monitor multiple resources involves integrating crucial aspects such as API security, AWS API Gateway, AI Gateway, and parameter rewrite/mapping. This efficient solution not only enhances resource management but also prepares an enterprise to leverage the benefits of cloud-native applications. The rapid deployment and management of APIs through APIPark further streamline this process. The focus on real-time data monitoring and efficient resource handling can significantly contribute to an organization’s agility in today’s fast-paced digital landscape.
8. References
- APIPark Documentation
- AWS API Gateway Documentation
- Go Documentation
- API Security Best Practices
By adapting these practices and leveraging robust structures like a dynamic informer, businesses can establish better monitoring systems tailored to their specific needs. Integrating AI solutions can lead to even more innovative approaches in resource management.