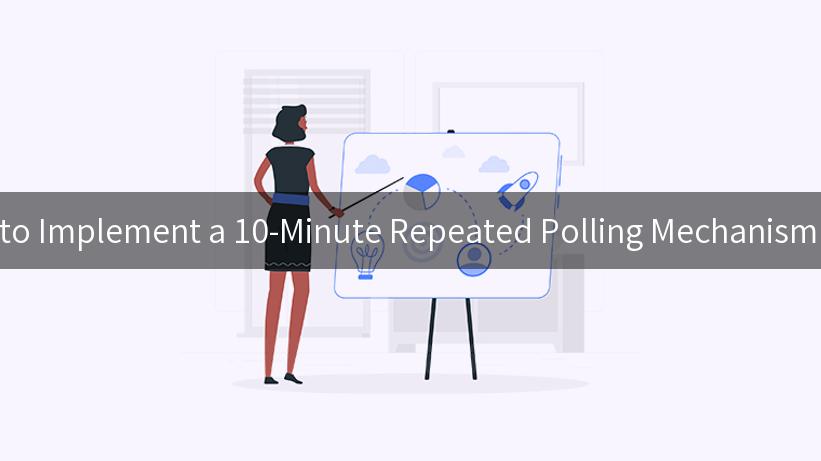
In today’s fast-paced and data-driven environment, the ability to efficiently interact with APIs is paramount. A polling mechanism enables applications to gather data from an API endpoint at predefined intervals. In this article, we will dive deep into how to implement a 10-minute repeated polling mechanism in C#. This mechanism can be particularly useful in scenarios where real-time data updates are not critical, but periodic data retrieval is necessary.
Additionally, we will explore the concepts of AI Gateway, MLflow AI Gateway, OpenAPI, and API Lifecycle Management within the context of our polling mechanism, enhancing our understanding of how to structure API interactions effectively.
Understanding Polling Mechanisms
Before we get into the implementation details, let’s clarify what polling is. Polling is a technique where an application makes repeated requests to a server at specified intervals. This can serve several purposes, from checking the status of a resource to gathering new data.
Different Types of Polling
- Basic Polling: The client sends requests at fixed intervals until it receives the desired response or timeout occurs.
- Long Polling: The client sends a request, and the server holds that request open until there is new information to send.
- Event-Driven Polling: The server pushes notifications to clients while they perform normal requests; they can then fetch new data based on those notifications.
In our case, we will employ basic polling to check an endpoint repeatedly for 10 minutes.
Setting Up Your Environment
Before we jump into the coding part, we need to ensure we have our environment ready.
- IDE: Use Visual Studio or Visual Studio Code for your C# development.
- .NET SDK: Ensure you have the .NET SDK installed.
- Libraries: For making HTTP requests, we will use
System.Net.Http
.
Implementation Steps
The following sections will guide you through the implementation of a 10-minute repeated polling mechanism in C#. We will break this down step by step.
Step 1: Create a New Console Application
Open your terminal or command prompt and create a new console project by executing:
dotnet new console -n PollingApp
cd PollingApp
Step 2: Add Required Namespaces
In your Program.cs
, import the necessary namespaces:
using System;
using System.Net.Http;
using System.Threading.Tasks;
using System.Threading;
Step 3: Set Up the Polling Logic
Below is the code that implements the repeated polling mechanism for 10 minutes. This will issue a request to a specified endpoint at one-minute intervals:
class Program
{
private static readonly HttpClient client = new HttpClient();
static async Task Main(string[] args)
{
string url = "http://api.example.com/data"; // Replace this with your API endpoint
TimeSpan pollingInterval = TimeSpan.FromMinutes(1);
TimeSpan totalPollingTime = TimeSpan.FromMinutes(10);
DateTime endTime = DateTime.UtcNow.Add(totalPollingTime);
while (DateTime.UtcNow < endTime)
{
try
{
var response = await CallEndpoint(url);
Console.WriteLine($"Response: {response}");
}
catch (Exception ex)
{
Console.WriteLine($"Error occurred: {ex.Message}");
}
// Wait for the polling interval
await Task.Delay(pollingInterval);
}
}
private static async Task<string> CallEndpoint(string url)
{
var response = await client.GetAsync(url);
response.EnsureSuccessStatusCode();
return await response.Content.ReadAsStringAsync();
}
}
Step 4: Running the Application
To run your console application, execute:
dotnet run
You should now see your application making requests to the specified URL every minute for 10 minutes.
Understanding API Interaction with MLflow and OpenAPI
AI Gateway and MLflow AI Gateway
As the demand for AI applications grows, platforms like AI Gateway and MLflow AI Gateway play an essential role in managing and integrating such services. These platforms allow you to create a seamless interface for your AI models, promoting usability and accessibility.
-
AI Gateway: This is a service layer facilitating access to various AI models. It abstracts the details of model integration, allowing developers to focus on building applications without worrying about the underlying complexity.
-
MLflow AI Gateway: MLflow serves as an open-source platform for managing the ML lifecycle, including experimentation, reproducibility, and deployment. The integration with an AI Gateway allows streamlined access to your ML models via RESTful APIs.
API Lifecycle Management
Effective management of the API lifecycle is crucial for ensuring API stability, reliability, and security.
Using tools like APIPark, you can achieve harmonized API lifecycle management, which encompasses:
- Design: Planning your API structure using OpenAPI standards.
- Develop: Building and testing the API endpoints.
- Deploy: Launching your API with proper version control.
- Monitor: Analyzing API performance and managing usage metrics.
- Retire: Gracefully deprecating old APIs, ensuring consumers transition smoothly.
Polling Management and Best Practices
When implementing polling techniques, consider the following best practices:
- Backoff Strategy: Introduce a backoff mechanism to minimize server load in case of failures. For instance, if a request fails, you might want to increase the wait time before the next attempt.
- Timeouts and Limits: Set sensible timeouts and limits to avoid running indefinitely.
- Efficient Data Handling: Only process or store data that changes. Use state checking to ignore unchanged responses.
Conclusion
In this article, we walked through the process of implementing a 10-minute repeated polling mechanism in C#. The provided example demonstrated how straightforward it is to retrieve data from an endpoint periodically. Furthermore, we explored how concepts like AI Gateway, MLflow AI Gateway, OpenAPI, and API Lifecycle Management significantly enhance API interactions.
By applying these principles and practices, you can build efficient, scalable applications that integrate seamlessly with various services. Polling can be a powerful tool in your toolkit when used correctly, enabling you to create more responsive and intelligent applications.
Sample Polling Configuration Table
Parameter |
Value |
Polling Interval |
1 Minute |
Total Polling Time |
10 Minutes |
API Endpoint |
http://api.example.com/data |
Response Format |
JSON |
APIPark is a high-performance AI gateway that allows you to securely access the most comprehensive LLM APIs globally on the APIPark platform, including OpenAI, Anthropic, Mistral, Llama2, Google Gemini, and more.Try APIPark now! 👇👇👇
Further Reading
For those interested in exploring more about API management and integration with AI services, consider checking:
- The official OpenAPI Specification
- Documentation for MLflow
Implementing a robust polling mechanism has never been easier, and the introduction of cutting-edge API management tools only makes it more accessible. Happy coding!
🚀You can securely and efficiently call the Gemni API on APIPark in just two steps:
Step 1: Deploy the APIPark AI gateway in 5 minutes.
APIPark is developed based on Golang, offering strong product performance and low development and maintenance costs. You can deploy APIPark with a single command line.
curl -sSO https://download.apipark.com/install/quick-start.sh; bash quick-start.sh
In my experience, you can see the successful deployment interface within 5 to 10 minutes. Then, you can log in to APIPark using your account.
Step 2: Call the Gemni API.