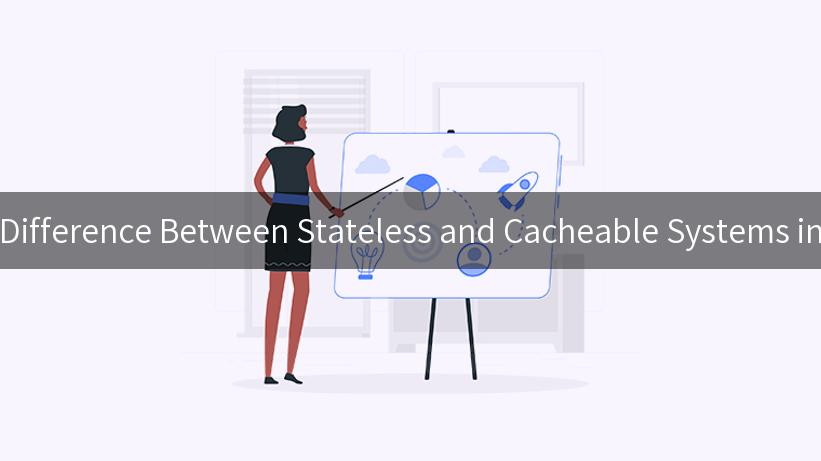
In the world of web development, the architecture of an application can make a significant difference in how it performs and scales. Two important concepts that developers often grapple with are stateless and cacheable systems. Understanding the distinctions between these two types of systems is essential for effective API design and implementation. In this article, we will explore the differences between stateless and cacheable systems, their implications for API security, version management, and how they relate to technologies like APISIX and the OpenAPI specification.
What are Stateless Systems?
Stateless systems are designed to treat each request independently. This means that every interaction with the client is handled without any knowledge of previous interactions. In stateless systems, all the necessary information is included in the request. This architecture has several benefits:
-
Scalability: Since no client state is maintained, stateless systems can easily scale horizontally. New servers can be added without needing to synchronize the state across them.
-
Simplicity: The stateless nature simplifies the server-side logic, as there is no need for managing or retaining client state.
-
Fault Tolerance: In a stateless system, if a server fails, another can take over without any loss of client data since no session state is stored.
Example of Stateless Systems
A popular example of a stateless system is the RESTful API architecture. In REST, each request must contain all the information the server needs to fulfill it. For instance, an API request made for user data would include all relevant authentication tokens and user identifiers.
Code Example: Stateless API Call
Here is a simple code example demonstrating a stateless API call using cURL:
curl --location 'http://api.example.com/users/1' \
--header 'Authorization: Bearer your_token_here'
In this example, every request to fetch user data must include a valid authorization token.
What are Cacheable Systems?
Cacheable systems, on the other hand, are designed to leverage caching mechanisms to store responses for future requests. By caching responses, these systems can significantly reduce load times and optimize performance. The cache acts as an intermediary between the client and server, allowing repeated requests for the same resource to be served quickly.
Key Advantages of Cacheable Systems
-
Performance Improvement: Caching reduces the time taken to fetch data, as it can be served from the cache rather than querying the database or backend service every time.
-
Reduced Load: Servers experience less load since repeated requests for the same resource do not have to be processed through the server stack.
-
Latency Reduction: Cacheable systems can significantly decrease latency for frequently accessed data, enhancing the user experience.
Characteristics of Cacheable Responses
Cacheable responses from an API are typically marked with specific HTTP headers such as Cache-Control
, Expires
, or ETag
. These headers signal to clients and intermediary caches how to treat the responses.
Example of a Cacheable Response
Consider the following response header from an API:
HTTP/1.1 200 OK
Cache-Control: max-age=3600
ETag: "v1"
Content-Type: application/json
{
"data": "This is cacheable data."
}
In this example, the Cache-Control
header indicates that the response can be cached for one hour.
Comparing Stateless vs. Cacheable Systems
Feature |
Stateless |
Cacheable |
Client State |
Not retained |
Retained until cache expires |
Scalability |
Highly scalable |
Scalable with caching strategy |
Server Load |
Balance on every request |
Reduced with cache hits |
Complexity |
Simpler implementation |
More complexity in cache management |
Performance |
Depends on server load |
Enhanced through caching |
When to Use Stateless vs. Cacheable Systems
The choice between using a stateless or cacheable system often depends on the specific requirements of your application. Here are some guidelines to consider:
API Security Considerations
In web development, API security is paramount. Whether employing a stateless or cacheable architecture, developers must ensure that APIs are secure from potential vulnerabilities.
Stateless API Security
Stateless systems tend to be easier to secure since each request is independent. Authentication tokens (like JWT) can be sent with each request, providing the necessary context without storing state on the server. However, managing token expiration and ensuring they are safely transmitted is critical.
Cacheable API Security
In cacheable systems, security can be more challenging. Since responses are cached, the data retrieved from the cache might become stale or vulnerable to unauthorized access. It’s essential to implement appropriate cache-control headers and regularly validate cached data against the backend to ensure that the data is still valid and secure.
API Version Management
Managing API versions is essential, especially in a production environment where breaking changes can disrupt client applications. Both stateless and cacheable systems require thoughtful strategies for API version management.
Strategies for Version Management
-
URL Versioning: Incorporate the version number into the URL (e.g., api.example.com/v1/resource
).
-
Header Versioning: Use custom headers to specify the desired API version.
-
Query Parameters: Allow clients to specify the version as a query parameter.
Comparison of Versioning Strategies
Strategy |
Pros |
Cons |
URL Versioning |
Clear and explicit; easy to manage. |
May lead to URL clutter; hard to maintain. |
Header Versioning |
More flexible; allows multiple versions to coexist. |
Can be less clear; requires clients to implement. |
Query Parameters |
Easy to implement; can be cached easily. |
URLs can become messy; lacking clarity about versions. |
Using APISIX for API Gateway Management
APISIX, an open-source API gateway, is an excellent tool for managing your APIs, particularly when dealing with stateless and cacheable systems. APISIX provides several features that facilitate API management:
- Traffic Management: Routing, load balancing, and traffic control to ensure efficient request handling.
- Security Plugins: Tools for authentication, rate limiting, and IP blacklisting to secure your APIs.
- Caching Capabilities: Built-in caching mechanisms to improve performance for cacheable APIs.
- Monitoring and Analytics: Provides detailed logging and statistics to understand API usage patterns.
Leveraging OpenAPI Specifications
The OpenAPI specification (formerly known as Swagger) provides a standard way to define your APIs. It allows developers to describe the operations, parameters, and responses of an API in a structured format.
The Benefits of Using OpenAPI
- Documentation: Automatically generate interactive API documentation that is easy to maintain.
- Client Generation: Create client SDKs for various platforms based on the OpenAPI definitions.
- API Validation: Ensure that your APIs adhere to the defined contract, reducing the chances of errors.
Conclusion
In summary, understanding the difference between stateless and cacheable systems is vital for effective web development. Each approach comes with its strengths, weaknesses, and appropriate use cases. Security considerations, API version management, and the right tools like APISIX and OpenAPI play pivotal roles in developing robust and secure applications.
Whether you are building a RESTful API that requires high scalability or a performance-sensitive application that benefits from caching, making informed decisions about architecture will impact your application’s success. By analyzing requirements and weighing the pros and cons of each approach, developers can design APIs that not only meet business needs but also delight users with performance and reliability.
APIPark is a high-performance AI gateway that allows you to securely access the most comprehensive LLM APIs globally on the APIPark platform, including OpenAI, Anthropic, Mistral, Llama2, Google Gemini, and more.Try APIPark now! 👇👇👇
Stay tuned as we delve deeper into each of these concepts in future articles, exploring best practices and real-world examples to help you master web API development.
🚀You can securely and efficiently call the 月之暗面 API on APIPark in just two steps:
Step 1: Deploy the APIPark AI gateway in 5 minutes.
APIPark is developed based on Golang, offering strong product performance and low development and maintenance costs. You can deploy APIPark with a single command line.
curl -sSO https://download.apipark.com/install/quick-start.sh; bash quick-start.sh
In my experience, you can see the successful deployment interface within 5 to 10 minutes. Then, you can log in to APIPark using your account.
Step 2: Call the 月之暗面 API.