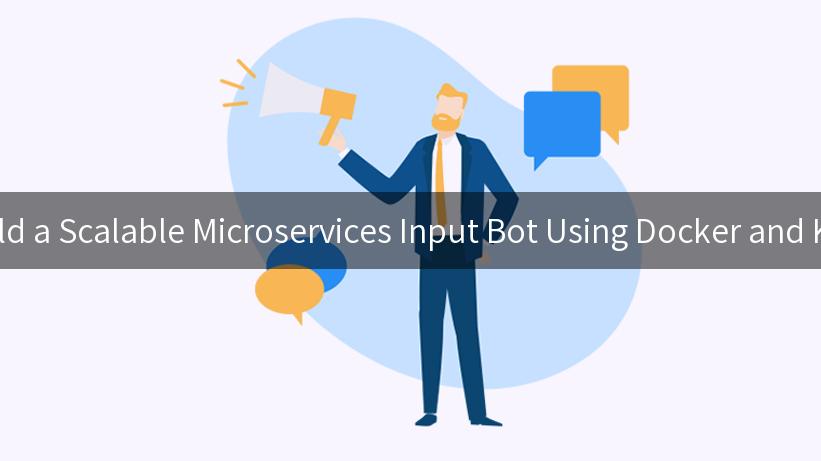
Creating a scalable microservices input bot can significantly enhance the efficiency and reliability of services, especially when integrating AI functionalities. This comprehensive guide will walk you through building such a bot utilizing technologies like Docker, Kubernetes, and AI-driven services. Additionally, we will touch on essential tools like Aisera LLM Gateway, API gateways, and API Exception Alerts to ensure safe operations and enable effective monitoring.
Understanding Microservices Architecture
Microservices architecture is an architectural style that structures an application as a collection of loosely coupled services. In the context of an input bot, this means each service is responsible for a specific functionality. This separation allows for easier maintenance, scaling, and deployment of individual services without affecting the entire system.
Advantages of Microservices
- Modularity: You can develop, update, and deploy each service independently.
- Scalability: Services can be scaled according to demand, allowing efficient resource allocation.
- Resilience: Failure in one service doesn’t necessarily affect others, enhancing the overall application’s reliability.
Key Components You’ll Need
To build a microservices input bot, you will need the following components:
- Docker: To containerize your applications and ensure consistent environments.
- Kubernetes: To orchestrate and manage your containers in a scalable manner.
- AI Services: Such as Aisera LLM Gateway, for enhanced functionalities.
- API Gateway: To provide a single entry point for your microservices and manage API traffic efficiently.
- Monitoring Tools: To set up API Exception Alerts and ensure the service operates smoothly.
Setting Up Your Development Environment
Prerequisites
Before starting the development process, ensure your environment is set up correctly:
- Install Docker: Ensure that Docker is running on your machine.
- Install Kubernetes: You can use Minikube for local development or set up a managed Kubernetes service like Google Kubernetes Engine (GKE).
- Familiarity with Git: Version control is essential for collaborative development.
- Code editor such as VSCode or IntelliJ.
Project Structure
Create a project directory that will house your microservices and configuration files.
mkdir microservices-input-bot
cd microservices-input-bot
Containerizing Your Microservices
Create a Sample Service
For demonstration purposes, create a simple Node.js application. Create a directory for your service:
mkdir input-service
cd input-service
Create a file named app.js
:
const express = require('express');
const app = express();
const PORT = process.env.PORT || 3000;
app.use(express.json());
app.post('/input', (req, res) => {
const userInput = req.body.input;
// Process the user input and return a response
res.json({ message: `Received: ${userInput}` });
});
app.listen(PORT, () => {
console.log(`Input service running on port ${PORT}`);
});
Create a Dockerfile
to containerize this service:
# Use Node.js image from the Docker Hub
FROM node:14
# Set working directory
WORKDIR /usr/src/app
# Copy package files
COPY package*.json ./
# Install dependencies
RUN npm install
# Copy service files
COPY . .
# Expose the port the app runs on
EXPOSE 3000
# Command to run the application
CMD ["node", "app.js"]
Build and Run Your Docker Container
Run the following command to build your Docker image:
docker build -t input-service .
Then, start the container using:
docker run -p 3000:3000 input-service
You should now see your input service running on http://localhost:3000/input
.
Deploying Your Microservices to Kubernetes
Create Kubernetes Configuration Files
To deploy your newly created microservice to Kubernetes, you need to create a deployment and service YAML configuration. Create a directory named k8s
in your project folder:
mkdir k8s
cd k8s
In the k8s
directory, create a file named input-service-deployment.yaml
:
apiVersion: apps/v1
kind: Deployment
metadata:
name: input-service
spec:
replicas: 3
selector:
matchLabels:
app: input-service
template:
metadata:
labels:
app: input-service
spec:
containers:
- name: input-service
image: input-service:latest
ports:
- containerPort: 3000
And create another file named input-service-service.yaml
:
apiVersion: v1
kind: Service
metadata:
name: input-service
spec:
type: ClusterIP
selector:
app: input-service
ports:
- port: 80
targetPort: 3000
Apply Your Kubernetes Configuration
Use the kubectl command to deploy your services and expose them through a service:
kubectl apply -f k8s/input-service-deployment.yaml
kubectl apply -f k8s/input-service-service.yaml
Accessing Your Service
To access your service externally for testing, you can use port forwarding:
kubectl port-forward service/input-service 8080:80
Now you can access your input service at http://localhost:8080/input
.
Integrating with Aisera LLM Gateway
To amplify the capabilities of your input bot, you can integrate AI services via the Aisera LLM Gateway. The Aisera LLM Gateway offers easy access to AI functionalities that can enhance user interaction and provide intelligent responses.
Setting Up Aisera LLM Gateway
- Sign Up/Login: First, obtain an account with Aisera and get the necessary API access.
- Configuration: Follow the API integration guide to configure your AI model and set up tokens.
Implement AI Services in Your Microservice
Update the /input
route in your app.js
to contact the Aisera LLM Gateway:
const axios = require('axios'); // Add axios for HTTP requests
app.post('/input', async (req, res) => {
const userInput = req.body.input;
const aiResponse = await axios.post('https://aisera.api/endpoint', {
input: userInput,
headers: {
'Authorization': `Bearer YOUR_AISERA_API_TOKEN`
}
});
res.json({ message: `AI Response: ${aiResponse.data.message}` });
});
This integration allows for intelligent responses depending on user inputs, combining the strengths of microservices architecture and AI capabilities.
Managing API Gateways
An API Gateway is essential for microservices architecture, providing routing, composition, and protocol translation. This management layer will also help enforce security policies, handle API Exception Alerts, and monitor service interactions.
Setting Up an API Gateway
You may choose popular tools like Kong, AWS API Gateway, or NGINX. For instance, if you opt for Kong, your configuration might look like this:
- Install Kong: Follow the installation instructions available on their official site.
- Create a Service:
curl -i -X POST http://localhost:8001/services/ \
--data 'name=input-service' \
--data 'url=http://input-service:3000'
- Create a Route:
curl -i -X POST http://localhost:8001/services/input-service/routes \
--data 'paths[]=/input'
This sets up an API gateway that routes requests from http://yourgateway:port/input
to your input service.
Monitoring and Exception Alerts
Monitoring an active microservices environment is critical for ensuring service reliability. Use tools like Prometheus and Grafana for effective monitoring and visualize performance metrics.
Setting Up Alerts
To set up API Exception Alerts, you can utilize Alertmanager (a component of Prometheus) to receive alerts for any irregularities in API usage.
- Alert Rules: Define alert rules in Prometheus configuration:
groups:
- name: api-alerts
rules:
- alert: HighErrorRate
expr: sum(rate(http_requests_total{status="500"}[5m])) by (service) > 0.05
for: 10m
labels:
severity: page
annotations:
summary: "High Error Rate Detected"
description: "Service {{ $labels.service }} is experiencing a high error rate."
- Integrate Alertmanager: Ensure your Alertmanager is configured to send messages to collaboration tools like Slack, email, or other channels for immediate notification.
Conclusion
Building a scalable microservices input bot using Docker and Kubernetes requires understanding microservices architecture, integrating AI services, managing API gateways, and monitoring performance. The incorporation of Aisera LLM Gateway, API Exception Alerts, and effective scaling strategies ensures that your input bot remains responsive and efficient.
By following this guide, you can create a robust and efficient microservices architecture that leverages advanced AI functionalities while maintaining high levels of reliability and scalability. For future reference and enhancements, explore different microservice design patterns, API management strategies, and monitoring solutions to adapt to growing business needs.
References
- Docker Documentation
- Kubernetes Documentation
- Aisera Documentation
- API Gateway Documentation
- Prometheus Documentation
- Grafana Documentation
APIPark is a high-performance AI gateway that allows you to securely access the most comprehensive LLM APIs globally on the APIPark platform, including OpenAI, Anthropic, Mistral, Llama2, Google Gemini, and more.Try APIPark now! 👇👇👇
This comprehensive exploration has provided you with the foundational knowledge to build a scalable microservices input bot using modern containerization and orchestration technologies. By leveraging AI capabilities and robust monitoring solutions, your input bot can serve as a vital component in any digital infrastructure. Happy coding!
🚀You can securely and efficiently call the gemni API on APIPark in just two steps:
Step 1: Deploy the APIPark AI gateway in 5 minutes.
APIPark is developed based on Golang, offering strong product performance and low development and maintenance costs. You can deploy APIPark with a single command line.
curl -sSO https://download.apipark.com/install/quick-start.sh; bash quick-start.sh
In my experience, you can see the successful deployment interface within 5 to 10 minutes. Then, you can log in to APIPark using your account.
Step 2: Call the gemni API.