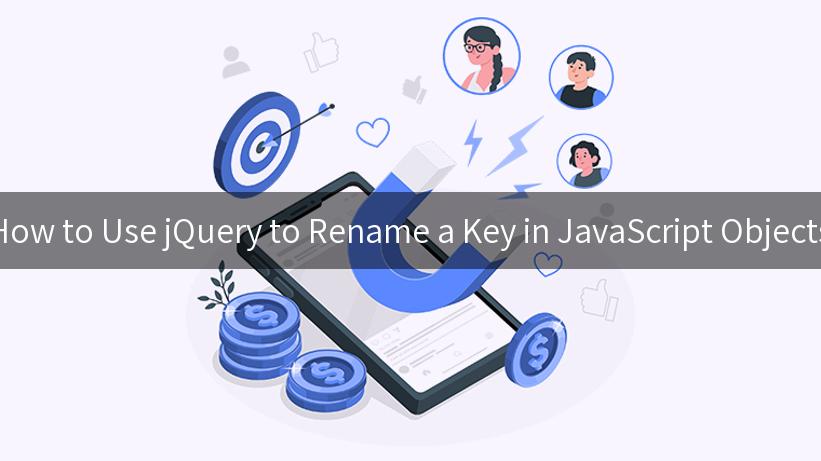
Renaming a key in JavaScript objects can be a frequent requirement in many web applications. In this article, we will delve into how we can use jQuery to handle this task efficiently. We will discuss practical examples and demonstrate the renaming of keys within nested objects, something that is particularly useful when dealing with complex data structures obtained from various APIs like those from APIPark or Kong. Additionally, we will touch on the concept of API Developer Portals and the integration of additional header parameters, enriching our understanding of API management and implementation from a frontend perspective.
Understanding JavaScript Objects
JavaScript objects are collections of key-value pairs, where each key is a string (also known as a property name) and the associated value can be any valid JavaScript data type. When working with objects, it may occasionally be necessary to rename a key. For example, when retrieving data from an API, the keys might not match your application’s needs.
Basic Object Syntax
Here’s a simple example of a JavaScript object:
var person = {
name: "Alice",
age: 25,
city: "New York"
};
In this object, name
, age
, and city
are keys, and their corresponding values are “Alice”, 25, and “New York”.
Why You Might Need to Rename an Object Key
There could be several reasons for renaming an object key:
- API Response Compatibility: If you receive data from an API that has keys that do not match your own conventions.
- Data Normalization: To ensure consistency in your application data structure.
- Code Readability: To make the object properties more intuitive for developers working on the same project.
When dealing with APIs, using a robust API Developer Portal, like APIPark, allows for effective management of APIs and related data structures. For instance, using Kong with APIPark, you can manage additional header parameters that might also require adjustments in the associated objects.
Using jQuery to Rename a Key
While JavaScript provides methods to rename object keys directly, jQuery can streamline this process. Here’s a step-by-step guide.
Step 1: Basic Syntax for Key Renaming
To rename a key, you can follow these steps:
- Create a new key with the desired name.
- Assign the value of the old key to the new key.
- Delete the old key.
Example: Renaming a Key
Let’s say you want to rename the city
key to location
in the person
object.
$(document).ready(function() {
var person = {
name: "Alice",
age: 25,
city: "New York"
};
// Renaming `city` to `location`
person.location = person.city; // Step 1: Assign old value to new key
delete person.city; // Step 2: Delete old key
console.log(person); // { name: 'Alice', age: 25, location: 'New York' }
});
Step 2: Renaming Keys in Nested Objects
Now, let’s see how we can handle more complex structures, such as nested objects. Imagine your API provides data in this format:
var employee = {
id: 101,
details: {
fullName: "Bob Smith",
department: "Engineering"
}
};
If you want to rename fullName
to name
, here’s how you would do it:
$(document).ready(function() {
var employee = {
id: 101,
details: {
fullName: "Bob Smith",
department: "Engineering"
}
};
// Renaming `fullName` to `name`
employee.details.name = employee.details.fullName; // Assign value to new key
delete employee.details.fullName; // Delete old key
console.log(employee); // { id: 101, details: { name: 'Bob Smith', department: 'Engineering' } }
});
Step 3: Using A Function to Rename Keys Dynamically
To make the renaming process more flexible, we can create a reusable function. This function will take the object, the old key, and the new key as parameters.
function renameKey(obj, oldKey, newKey) {
if (oldKey in obj) {
obj[newKey] = obj[oldKey];
delete obj[oldKey];
}
}
// Usage
$(document).ready(function() {
var student = {
studentId: 202,
details: {
fullName: "Jane Doe",
major: "Computer Science"
}
};
renameKey(student.details, 'fullName', 'name');
console.log(student); // { studentId: 202, details: { name: 'Jane Doe', major: 'Computer Science' } }
});
Implementing in Real-Life Scenarios
This technique of renaming keys is particularly useful when dealing with data from APIs. When integrating with platforms such as APIPark or Kong, you may wish to remap API response data to your preferred format.
Example: Using APIPark Data
When calling an API from APIPark, you may retrieve data that needs slight modifications:
$.ajax({
url: 'http://api.apipark.com/getData',
method: 'GET',
success: function(response) {
// Assume response contains objects with keys that need renaming
// response could be like: [{ user_name: "Alice" }, { user_name: "Bob" }]
response.forEach(function(item) {
renameKey(item, 'user_name', 'name');
});
console.log(response); // [{ name: "Alice" }, { name: "Bob" }]
},
error: function(error) {
console.log('Error fetching data', error);
}
});
Avoiding Common Pitfalls
When renaming keys, ensure you:
- Check for the Key’s Existence: Make sure the key you wish to rename actually exists in the object to avoid potential errors or unexpected behavior.
- Be Mindful of Nested Structures: When working with nested objects, remember that you must handle nested properties accordingly.
- Use Meaningful Names: Always choose key names that make sense for the data they represent.
Conclusion
Manipulating JavaScript objects by renaming keys can streamline work with various data sources, especially when integrating APIs through platforms like APIPark. By leveraging jQuery, you can simplify the renaming process, making your code cleaner and more efficient.
To summarize, we:
- Discussed the need for renaming keys in JavaScript objects.
- Provided examples and use cases for practical application.
- Introduced a function for dynamic renaming.
Now you’re equipped to manage object keys effectively! Happy coding!
APIPark is a high-performance AI gateway that allows you to securely access the most comprehensive LLM APIs globally on the APIPark platform, including OpenAI, Anthropic, Mistral, Llama2, Google Gemini, and more.Try APIPark now! 👇👇👇
Additional Resources
Resource |
Description |
APIPark Documentation |
Access comprehensive API integration guides and quick setup. |
jQuery Documentation |
Official jQuery documentation for in-depth method references. |
Kong Documentation |
Learn how to manage APIs and additional parameters effectively. |
Integrating tools and practices, along with adapting this simple key renaming technique, creates a more robust application development life cycle. Now it’s time to put what you’ve learned into practice!