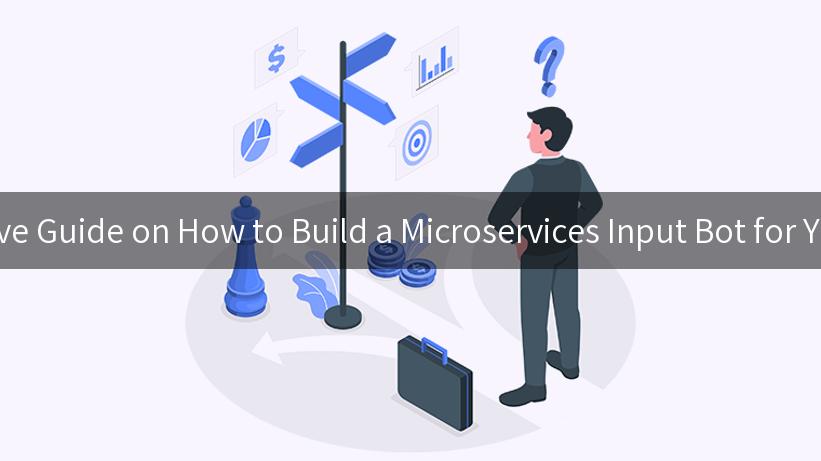
In today’s rapidly evolving tech landscape, building applications using microservices architecture has become a predominant practice. Microservices allow for the development of applications as a suite of small, independently deployable services that each run a unique process. This article will provide a comprehensive guide on how to build a microservices input bot for your application, specifically leveraging tools like APIPark and Cloudflare for optimized performance and seamless routing.
What is a Microservices Input Bot?
Before diving into the technical details, let us define what exactly a microservices input bot is. A microservices input bot is designed to handle user inputs and data submissions from various sources, manipulating and routing them to the appropriate microservices accordingly. This can significantly improve data handling efficiency and streamline communication between different parts of your application.
The integration of services through a gateway, such as APIPark, enhances the robustness of your bot, while tools like Cloudflare ensure secure and efficient routing.
Advantages of Building a Microservices Input Bot
- Improved Scalability: Microservices can scale independently, allowing you to allocate resources precisely where they are needed without affecting other services.
- Enhanced Maintainability: Smaller codebases are easier to update and maintain as opposed to monolithic applications.
- Increased Deployment Speed: Microservices can be deployed faster since changes in one service do not necessitate redeploying the entire application.
- Failure Isolation: Issues in a single service do not bring down the whole application.
How to Build a Microservices Input Bot
Now that we have established the importance of a microservices input bot, let’s dive into the detailed steps of how to construct one.
Step 1: Setting Up Your Environment
Before you proceed with coding, ensure you have a conducive environment set up.
- Install APIPark: This acts as the API gateway for managing your microservices.
bash
curl -sSO https://download.apipark.com/install/quick-start.sh; bash quick-start.sh
- Setup Cloudflare Account: Sign up for a Cloudflare account. This will be used to provide a secure gateway and manage DNS records.
The following table summarizes the initial setup requirements:
Tool |
Purpose |
Installation Command |
APIPark |
Manage API gateways |
curl -sSO https://download.apipark.com/install/quick-start.sh; bash quick-start.sh |
Cloudflare |
Provide security and routing |
Sign up on Cloudflare |
Step 2: Define the Microservices
Identify the functionality your input bot will handle:
- Data Validation Service
- User Input Processing Service
- Notification Service
Each of these functions will be implemented as a separate microservice, which improves performance and encapsulation.
Step 3: Implement the Input Bot Logic
You can create a simple input bot using Python, Flask, or Node.js. Below, you’ll find an example using Flask:
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route('/input', methods=['POST'])
def handle_input():
data = request.json
# Logic for processing input
# This could include validation, storing to a database, etc.
return jsonify({"message": "Input received", "data": data}), 200
if __name__ == '__main__':
app.run(port=5000)
This example demonstrates a basic Flask app that receives input data via a POST request.
Step 4: Integrating Cloudflare
After creating your microservice to handle input, set up Cloudflare to manage the traffic and provide security. You will need to:
- Set Up DNS Records: Point your domain to Cloudflare’s DNS.
- Create Page Rules: Set rules to cache certain pages or APIs to optimize speed.
- Enable SSL: For secure transmission of data.
Step 5: Configure Routing with APIPark
Now go back to your APIPark interface and configure the routing rules. Here’s how:
- Create an Application: Define an application that needs to call your services.
- Define Routes: Set up routing rules that direct API calls to your defined microservices.
- API Token: Generate and secure API tokens to control access.
Utilizing `
APIPark is a high-performance AI gateway that allows you to securely access the most comprehensive LLM APIs globally on the APIPark platform, including OpenAI, Anthropic, Mistral, Llama2, Google Gemini, and more.Try APIPark now! 👇👇👇
` here to add further details about advanced routing configurations, such as designing a failover path for high availability.
Step 6: Test Your Bot
Testing your input bot is crucial before deployment. Use tools like Postman or curl to send sample inputs:
curl --location 'http://localhost:5000/input' \
--header 'Content-Type: application/json' \
--data '{
"user": "test_user",
"input": "Hello, how can I help you?"
}'
Conclusion
Building a microservices input bot for your application using APIPark and Cloudflare is a strategic move to enhance performance and maintainability. By following the steps outlined in this guide, you can ensure that your application is prepared to handle user inputs efficiently, securely, and scalably.
Final Thoughts
As technology continues to innovate and evolve, the demand for microservices architecture will only grow. Leveraging tools such as APIPark and Cloudflare will play a critical role in developing robust applications capable of meeting this demand. Don’t hesitate to experiment with various services and configurations to tailor your microservices input bot to your specific needs.
Embrace the future of application development with microservices; it’s not just a trend but a shift towards more agile and resilient systems.
This comprehensive guide aims at providing you with all the necessary information to successfully build a microservices input bot while keeping usability and security at the forefront.