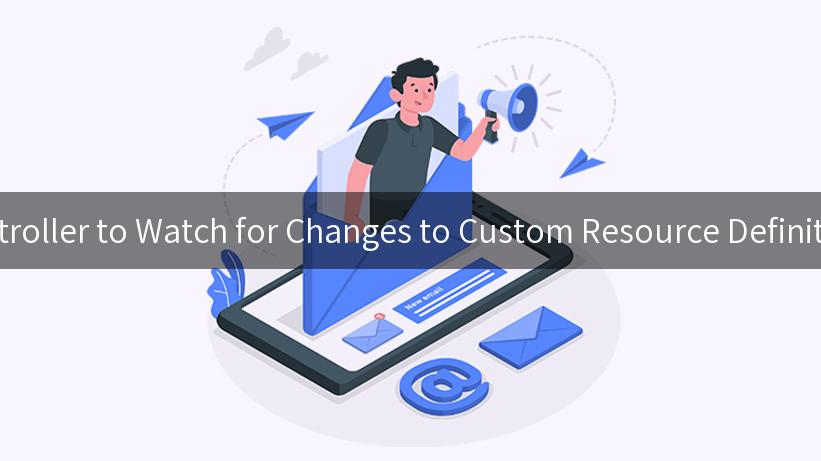
Kubernetes has revolutionized the way we deploy and manage applications in the cloud. With its powerful manipulation of resources, Kubernetes allows developers to extend its functionality using Custom Resource Definitions (CRDs). In this article, we will delve into how to implement a controller that watches for changes to these CRDs in Kubernetes. Additionally, we will explore key concepts such as the AI Gateway, IBM API Connect, and API Version Management.
Understanding Custom Resource Definitions (CRDs)
Custom Resource Definitions, or CRDs, are extensions of the Kubernetes API. They allow you to define your own resource types, effectively allowing Kubernetes to acknowledge them just like native resources (e.g., Pods, Services). This adds immense flexibility, letting you integrate Kubernetes with various external systems and workflows.
Why Watch for Changes in CRDs?
Monitoring changes to CRDs is crucial in various scenarios such as:
- Automated Workflows: Triggering actions whenever a CRD is created, updated, or deleted.
- Integration Notifications: Informing external systems or services about the state changes of Kubernetes resources.
- Seamless Management: Ensuring the system behaves as expected under dynamic conditions.
By implementing a controller that monitors these changes, developers can automate impacts that result from resource state changes and enhance their system’s responsiveness.
Setting Up Your Kubernetes Environment
Before we dive into controller implementation, ensure you have a Kubernetes environment up and running. You will need kubectl
to interact with your cluster and create the necessary resources. If you’re new to Kubernetes, you can set up a local environment using Minikube or a cloud provider of your choice.
Installing the CRD
First, let’s create a simple CRD. You can define a CRD manifest like so:
apiVersion: apiextensions.k8s.io/v1
kind: CustomResourceDefinition
metadata:
name: myresources.example.com
spec:
group: example.com
versions:
- name: v1
served: true
storage: true
schema:
openAPIV3Schema:
type: object
properties:
spec:
type: object
properties:
name:
type: string
scope: Namespaced
names:
plural: myresources
singular: myresource
kind: MyResource
shortNames:
- mr
You can apply this CRD using the following command:
kubectl apply -f myresource-crd.yaml
Creating the Controller
A controller in Kubernetes continuously watches for changes in resources and makes adjustments accordingly. Below, we will create a simple custom controller to monitor our CRD.
Install Dependencies
If you are building your own controller, you may want to use the Operator SDK or Kubebuilder. They provide boilerplate code to help you get started quickly. You can install the Operator SDK via:
brew install operator-sdk
Implementing the Controller Logic
Let’s create a controller that watches single events add
, update
, and delete
for our MyResource
CRD:
- Create a new controller project:
bash
operator-sdk init --domain example.com --repo github.com/example/my-controller
cd my-controller
- Create a new API:
bash
operator-sdk create api --group example --version v1 --kind MyResource --resource --controller
- Write the Controller Logic: Open up the
controllers/myresource_controller.go
file to modify the reconcile function.
func (r *MyResourceReconciler) Reconcile(ctx context.Context, req ctrl.Request) (ctrl.Result, error) {
log := r.Log.WithValues("myresource", req.NamespacedName)
// Fetch the MyResource instance
myResource := &examplev1.MyResource{}
err := r.Get(ctx, req.NamespacedName, myResource)
if err != nil {
log.Error(err, "unable to fetch MyResource")
return ctrl.Result{}, client.IgnoreNotFound(err)
}
// Place your business logic here.
log.Info("Reconciling MyResource", "Spec", myResource.Spec)
// Update your status or take action based on state changes.
return ctrl.Result{}, nil
}
Register the Controller
You need to register your controller with the manager so that it can start watching the resources:
if err = (&controllers.MyResourceReconciler{
Client: mgr.GetClient(),
Log: log.WithName("controllers").WithName("MyResource"),
Scheme: mgr.GetScheme(),
}).SetupWithManager(mgr); err != nil {
log.Error(err, "unable to create controller", "controller", "MyResource")
os.Exit(1)
}
Deploying Your Controller
With the controller implemented and testing complete, it’s now time to deploy it. Build the Operator image:
make docker-build docker-push IMG=<your-controller-image>
Then deploy the operator to your Kubernetes cluster:
make deploy IMG=<your-controller-image>
From this point, your controller will start watching for changes to your CRD resources.
Benefits of Using AI Gateway and IBM API Connect
When dealing with microservices architecture, especially with Kubernetes, you may want to use an AI Gateway or API Management tools such as IBM API Connect. These tools simplify API Version Management and help in seamless communication between services, providing additional layers of security and monitoring functionalities.
The Role of AI Gateway
An AI Gateway facilitates the interaction between client requests and backend services by managing traffic with features such as:
- Rate Limiting: Prevent excessive API calls to your services.
- Security: Implement OAuth, JWT tokens for securing APIs.
- Analytics: Track usage patterns and performance metrics.
Integrating with IBM API Connect
IBM API Connect provides a comprehensive solution for managing APIs, enabling organizations to create, manage, and secure APIs for both internal and external consumption. It comes equipped with features such as:
- Self-service Portal: Allows developers to register and consume APIs easily.
- Version Management: Efficient management of different versions of your APIs.
- Analytics Dashboard: Monitor API performance and response times.
Conclusion
Implementing a controller to watch for changes to Custom Resource Definitions (CRD) in Kubernetes enhances your ability to automate, respond, and manage your resources effectively. By combining this functionality with tools like AI Gateway and IBM API Connect, organizations can expand their microservices architecture, streamline API interactions, and improve overall system reliability.
By understanding and leveraging these advanced features, developers can ensure their Kubernetes deployments are future-proof, scalable, and maintainable. The seamless integration of AI services with Kubernetes infrastructure opens the door to building intelligent cloud-native applications.
> APIPark is a high-performance AI gateway that allows you to securely access the most comprehensive LLM APIs globally on the APIPark platform, including OpenAI, Anthropic, Mistral, Llama2, Google Gemini, and more.Try APIPark now! 👇👇👇
In this article, we have laid out a comprehensive guide on building a controller to watch for changes in CRDs, while also discussing the benefits of integrating with API management solutions. If you have any questions or need further assistance with implementing these technologies, feel free to reach out or explore the provided resources on the official Kubernetes documentation.
Additional Resources
Topic |
Resource Link |
Kubernetes Documentation |
Kubernetes Docs |
Official CRD Guide |
CRD Documentation |
Operator SDK Documentation |
Operator SDK Docs |
IBM API Connect |
IBM API Connect |
This has concluded our exploration into how to implement a controller for CRDs in Kubernetes, as well as the utility of combining this approach with AI and API Management tools.
🚀You can securely and efficiently call the Wenxin Yiyan API on APIPark in just two steps:
Step 1: Deploy the APIPark AI gateway in 5 minutes.
APIPark is developed based on Golang, offering strong product performance and low development and maintenance costs. You can deploy APIPark with a single command line.
curl -sSO https://download.apipark.com/install/quick-start.sh; bash quick-start.sh
In my experience, you can see the successful deployment interface within 5 to 10 minutes. Then, you can log in to APIPark using your account.
Step 2: Call the Wenxin Yiyan API.