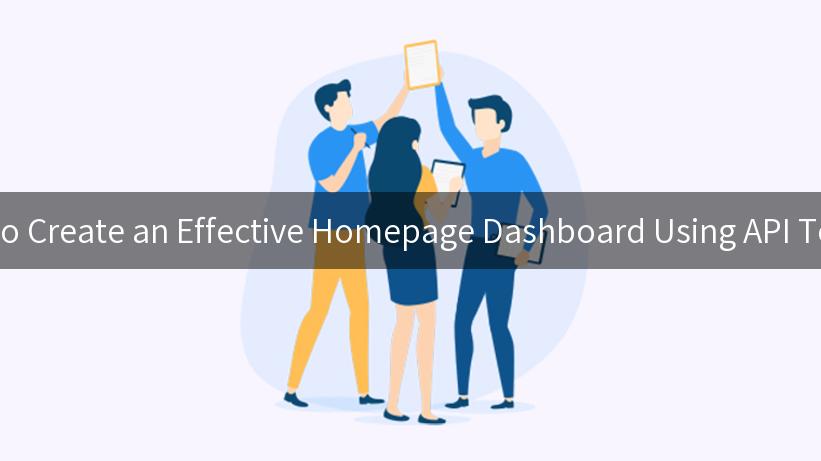
Creating an effective homepage dashboard is integral for visualizing important data and ensuring that users have quick access to the information they need. In this guide, we’ll explore how to create a compelling homepage dashboard using API tokens, focusing on emerging technologies such as AI security and integrating services like Wealthsimple LLM Gateway while managing API exception alerts.
Understanding API Tokens
An API Token is like a password that allows one software application to interact with another securely. It ensures that requests sent to the API are valid and authenticated. API tokens vastly improve security, aid in logging, and enable the controlled flow of data between multiple applications.
Key Benefits of Using API Tokens
- Enhanced Security: API tokens authenticate application requests without exposing sensitive credentials.
- Granular Access Control: Tokens can be configured to grant different levels of access to different parts of the application.
- Efficient Usage: By limiting access, tokens reduce the load on the server and are easier to manage than traditional username/password logins.
Benefits of API Tokens |
Description |
Enhanced Security |
Secure application without exposing sensitive information. |
Granular Access Control |
Control what data and services users can access. |
Efficient Usage |
Manage server load and authentication easily. |
Step-by-Step Guide to Creating Your Homepage Dashboard
Now that we understand API tokens, let’s dive into the step-by-step process of creating an effective homepage dashboard.
Step 1: Define Your Dashboard Objectives
Before starting the technical setup, determine what information and features your dashboard will display. Consider factors such as:
- Target Audience: Who will use the dashboard?
- Data Sources: What APIs will you utilize?
- Key Metrics: What key performance indicators (KPIs) will you include?
Step 2: Gather Your API Tokens
For this tutorial, we’ll be using Wealthsimple LLM Gateway as our primary API service. This service provides flexible and strong AI functionalities that can be easily integrated into your dashboard.
To gather the API tokens:
- Sign up for the Wealthsimple LLM service at the official site.
- Access the API section and generate your unique API token.
- Note down your token safely for use in API calls.
Step 3: Setup Your Dashboard Environment
You can set up a simple web environment using HTML, CSS, and JavaScript. Here’s an example of a basic structure:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Homepage Dashboard</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<header>
<h1>Homepage Dashboard</h1>
</header>
<main>
<section id="api-data">
<p>Loading...</p>
</section>
</main>
<script src="script.js"></script>
</body>
</html>
Here, we have a simple web page structure ready to display the data we will pull from the API.
Step 4: Fetch Data Using API Tokens
Now it’s time to fetch data from the Wealthsimple LLM Gateway using the API token. Below is a JavaScript code snippet that demonstrates how to make an API call:
const fetchDashboardData = async () => {
const apiToken = 'your_api_token_here';
const url = 'https://api.wealthsimple.com/v1/dashboard'; // Replace with actual API URL
try {
const response = await fetch(url, {
method: 'GET',
headers: {
'Authorization': `Bearer ${apiToken}`,
'Content-Type': 'application/json',
},
});
if (!response.ok) {
throw new Error('API Error');
}
const data = await response.json();
document.getElementById('api-data').innerHTML = JSON.stringify(data);
} catch (error) {
console.error('Error fetching data:', error);
document.getElementById('api-data').innerHTML = 'Error fetching data';
}
};
fetchDashboardData();
In this code snippet, replace your_api_token_here
with the actual token you obtained during setup. This function fetches data, handles errors, and displays the fetched data on the dashboard.
Step 5: Implement Alerts for API Exceptions
To enhance the dashboard experience, implement API Exception Alerts. This will notify you when an API call fails, ensuring high reliability of your application.
const checkApiStatus = (response) => {
if (!response.ok) {
alert('API Exception: ' + response.statusText);
}
};
Incorporate the checkApiStatus
function within the fetchDashboardData
function to monitor API calls effectively.
Step 6: Style Your Dashboard
Make your dashboard visually appealing by applying styles through CSS. Here is an example:
body {
font-family: Arial, sans-serif;
background-color: #f4f4f4;
}
header {
background: #35424a;
color: #ffffff;
padding: 10px 0;
text-align: center;
}
main {
padding: 20px;
}
#api-data {
background: #ffffff;
padding: 20px;
border-radius: 5px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
Step 7: Test Your Dashboard
Once everything is set up, test your homepage dashboard to ensure all features function as intended. Make API calls to validate that data fetches correctly and observe how alerts respond to any issues.
Step 8: Continuous Improvement
As you deploy your homepage dashboard, gather feedback from users to find areas for improvement. Updating your dashboard based on user experiences will keep it effective and relevant.
APIPark is a high-performance AI gateway that allows you to securely access the most comprehensive LLM APIs globally on the APIPark platform, including OpenAI, Anthropic, Mistral, Llama2, Google Gemini, and more.Try APIPark now! 👇👇👇
Conclusion
Creating an effective homepage dashboard using API tokens is an excellent way to harness collected data in a meaningful way. By leveraging services like Wealthsimple LLM Gateway, we can access robust functionalities. Implementing features like API exception alerts ensures a reliable experience for users, maintaining the integrity and performance of our dashboard. With continuous improvement and a focus on user feedback, your homepage dashboard can evolve into a powerful tool for data visualization.
By following the steps outlined in this guide, anyone can build a functional and secure homepage dashboard that effectively utilizes APIs and enhances the overall user experience.