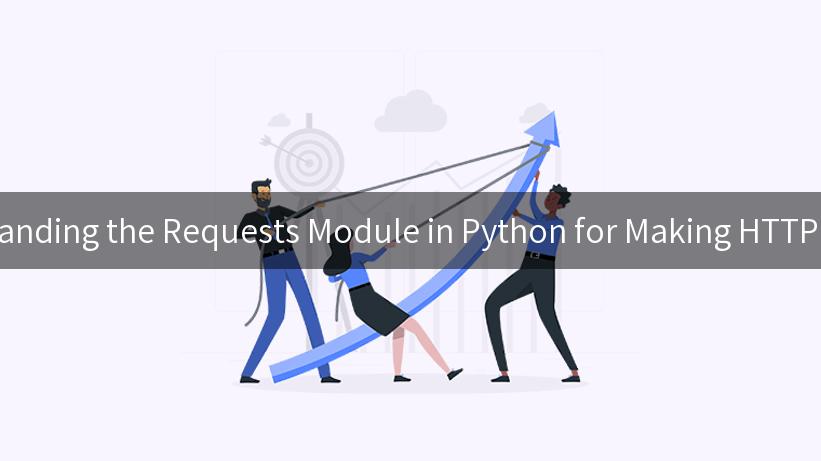
In today’s digital landscape, the ability to send and receive data over the internet using HTTP requests is central to building functional and efficient applications. For Python developers, the requests
module offers a straightforward and user-friendly way to handle HTTP operations. In this article, we will explore the functionality of the requests
module, its capabilities, and how it can be effectively used alongside an AI Gateway, MLflow AI Gateway, and Oauth 2.0 for secure and efficient API calls.
Introduction to HTTP Requests
Before delving into the requests
module, it is essential to understand what HTTP requests are. HTTP (HyperText Transfer Protocol) is the foundation of data communication on the web. It consists of methods, or “verbs,” such as GET, POST, PUT, DELETE, etc., each serving a specific purpose for communication with a server.
Types of HTTP Requests
- GET: This method is used to retrieve data from a server. It doesn’t modify data on the server.
- POST: This method is used to send data to the server to create or update resources.
- PUT: This method is used for updating existing resources.
- DELETE: This method is used for deleting specified resources.
Using the requests
module in Python, developers can easily handle these HTTP methods, making it a vital tool in the toolkit of anyone working with APIs.
What is the Requests Module?
The requests
module in Python is a powerful library designed to facilitate HTTP requests. It allows developers to easily interact with RESTful web services and APIs. The module abstracts away many of the complexities of sending HTTP requests, parsing responses, and handling sessions, providing a cleaner and more pythonic interface for these operations.
Key Features of the Requests Module
- Ease of Use: The
requests
library makes it simple to send HTTP requests with minimal code.
- Session Management: It maintains a session state, allowing multiple requests to persist settings across them.
- Automatic Content Decoding: Responses are decoded automatically; you do not need to worry about encoding type.
- Custom Headers: Developers can easily add custom headers to their requests.
- Timeout Handling: It allows specifying timeout constraints to avoid hanging during slow network calls.
Installation
Before using the requests
module, you need to install it. Use the following command to install it via pip:
pip install requests
Basic Usage
Here is a simple example of using the requests
module to make a GET request:
import requests
response = requests.get('https://api.example.com/data')
if response.status_code == 200:
print(response.json())
else:
print(f"Error: {response.status_code}")
In this example, a GET request is sent to a specified URL, and the response’s status code is checked. If the request is successful, the JSON data returned by the server will be printed.
Making Query Parameters
Sometimes, it is necessary to send query parameters along with an HTTP request. The requests
module handles this quite well using the params
argument. Here’s how you can do it:
import requests
params = {
'query': 'AI Gateway',
'page': 2
}
response = requests.get('https://api.example.com/search', params=params)
print(response.url) # This will print the complete URL with query parameters
print(response.json())
In the code above, we send the params
dictionary that defines the parameters to be sent with the GET request. The requests
library automatically appends these parameters to the URL.
Sending Data with POST Requests
To send data to a server, a POST request is used. You can send data through various formats, such as URL-encoded data, JSON, or multipart files. Let’s see how to send JSON data with a POST request:
import requests
data = {
'username': 'example_user',
'password': 'example_pass'
}
response = requests.post('https://api.example.com/login', json=data)
if response.status_code == 200:
print("Login successful!", response.json())
else:
print("Login failed!", response.status_code)
In this example, we send a JSON payload to the server using the json
argument, allowing for a clean and compact method of encoding the data.
Integrating Oauth 2.0 with Requests
When working with APIs that require authentication, Oauth 2.0 is a common framework used to secure data transactions. The requests
module simplifies the authentication process. Here is an example of how to use Oauth 2.0:
import requests
from requests_oauthlib import OAuth2Session
# Set up OAuth 2.0 parameters
client_id = 'YourClientID'
client_secret = 'YourClientSecret'
token_url = 'https://api.example.com/oauth/token'
api_url = 'https://api.example.com/secure-data'
# Obtain an access token
oauth = OAuth2Session(client_id)
token = oauth.fetch_token(token_url=token_url,
client_secret=client_secret)
# Now make authenticated requests
response = oauth.get(api_url)
print(response.json())
In this example, we use the requests_oauthlib
library, which extends the capabilities of the requests
module to handle Oauth 2.0. After obtaining an access token, we can make secure API calls using our authenticated session.
Leveraging AI with The Requests Module
With the rise of AI technologies, many applications now require integration with AI services. The requests
module is often employed to interact with AI gateways and platforms such as the MLflow AI Gateway.
Example: Calling an AI Service
Here’s an example of how to call an AI service using the requests
module:
import requests
url = "http://mlflow-ai-gateway/api/predict"
headers = {
"Authorization": "Bearer your_token_here",
"Content-Type": "application/json"
}
data = {
"input_data": [
# Your input data for the AI model
]
}
response = requests.post(url, headers=headers, json=data)
if response.status_code == 200:
print("AI response:", response.json())
else:
print("Error calling AI service:", response.status_code)
In this example, we send a POST request to an MLflow AI Gateway API with an authorization header and a JSON payload containing the input data. The response can then be parsed to retrieve the predictions or results from the AI model.
APIPark is a high-performance AI gateway that allows you to securely access the most comprehensive LLM APIs globally on the APIPark platform, including OpenAI, Anthropic, Mistral, Llama2, Google Gemini, and more.Try APIPark now! 👇👇👇
Debugging HTTP Requests
Debugging HTTP requests can sometimes be tricky. The requests
module provides built-in logging, which can be quite helpful. Here’s how you can enable logging for your HTTP requests:
import logging
import requests
# Enable logging
logging.basicConfig(level=logging.DEBUG)
response = requests.get('https://api.example.com/data')
By setting the logging level to DEBUG, you can see the requests being made, including headers and response codes, helping you trace issues more effectively.
Conclusion
The requests
module is a powerful and essential tool for Python developers when it comes to making HTTP queries. Whether you are interacting with web services or integrating AI functionalities through AI gateways like MLflow, the ease of use and flexibility of the requests
library streamline the process of API integration.
In the ever-evolving landscape of software development, understanding the capabilities of the requests
module, combined with knowledge of authentication protocols such as Oauth 2.0, ensures you can build robust applications that securely interact with various services.
The combination of powerful libraries, such as requests
, and the implementation of AI services create exciting potentials for innovation and efficiency in application development. So start integrating your applications with HTTP queries today!
Summary Table
Feature |
Description |
Ease of Use |
Allows simple HTTP requests with concise syntax. |
Session Management |
Maintains state across requests, making it easy to manage sessions. |
Automatic Decoding |
Responses are decoded according to content type automatically. |
Custom Headers |
Allows easy addition of headers for requests. |
Oauth Integration |
Simplifies secure API requests with Oauth 2.0 authentication. |
Using the requests
module for making HTTP queries is essential for achieving seamless service integrations and fulfilling modern application requirements. Embrace it to enhance your development workflow!
🚀You can securely and efficiently call the Anthropic API on APIPark in just two steps:
Step 1: Deploy the APIPark AI gateway in 5 minutes.
APIPark is developed based on Golang, offering strong product performance and low development and maintenance costs. You can deploy APIPark with a single command line.
curl -sSO https://download.apipark.com/install/quick-start.sh; bash quick-start.sh
In my experience, you can see the successful deployment interface within 5 to 10 minutes. Then, you can log in to APIPark using your account.
Step 2: Call the Anthropic API.